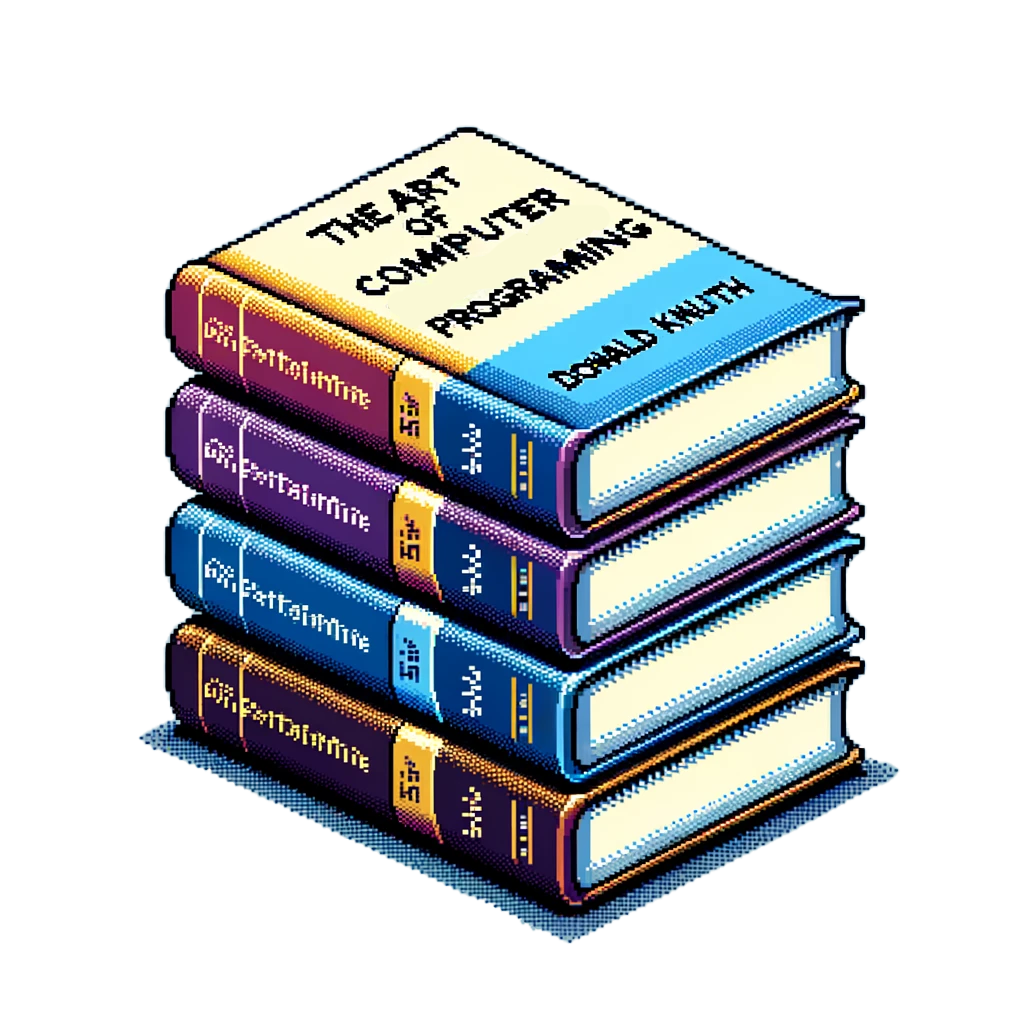
src Language
warning
This very much exists so I don't have to setup the open-source infrastructure after open-sourcing the project. There are lots of moving parts to the documentation, like the rustdocs is generated and included in the book. Also There is a IDE of sorts in playground and a language server that is used to provide the IDE.
src
is a domain specific language for manipulating source code and building, progressively distiributed apps or PDA.
It draws a lot of inspiration from Effekt and Koka languages.
src
is main aim is to provide a gradually distributed programming
environment for building software.
It tries to achive these goals by providing a thin veneer over the operating systems libc
or equivalent by treating the syscalls to the operating system as effects.
Therefore the operating system becomes the effect handler for the execution environment.
use { host } from std
effect Make: async + throws + execs + reads + writes {
catch() [throws]
await<T>(f: Future<T>) [async, throws] -> T
exec(arg0: string, args: stringvec) [Make] -> i32
}
struct Local {
host: host
}
impl Make for Local {
fn catch(self) [throws] {
}
fn await<T>(f: Future<T>) [async, trhows] -> T {
yield()
}
fn exec(self, arg0: string, args: vec<string>) [Vm] -> i32 {
self.host.read("jobserver").await
if self.host.exec(arg0, args) {
raise(1)
}
}
}
Acknowledgements
Building upon the incredible work of the Rust community and many others, src would not be possible without the following projects:
Language Specification
use super::ast::*;
use lalrpop_util::ErrorRecovery;
use crate::lexer::{Token, Location};
use crate::lexer::Word;
use crate::Db;
use super::span::Spanned;
use crate::span;
#[LALR]
grammar<'input, 'err>(errors: &'err mut Vec<ErrorRecovery<Location, Token<'input>, &'static str>>, db: &dyn Db);
extern {
type Location = Location;
enum Token<'input> {
// Operators
"|" => Token::Pipe, // |
"&" => Token::Ampersand, // &
";" => Token::Semicolon, // ;
"=" => Token::Equals, // =
// Redirections
"<" => Token::LessThan, // <
">" => Token::GreaterThan, // >
// Identifiers
// "param" => Variable::Parameter(<&'input str>), // var
// "param_default" => Variable::ParameterDefault(<&'input str>, <&'input str>), // var = value
// "positional_param" => Variable::PositionalParameter(<usize>), // $var
// Literals
"true" => Token::Word(Word::True), // true
"none" => Token::Word(Word::None), // none
"false" => Token::Word(Word::False), // false
"null" => Token::Word(Word::Null), // null
"fn" => Token::Word(Word::Fn), // fn
"if" => Token::Word(Word::If), // if
"else" => Token::Word(Word::Else), // else
"match" => Token::Word(Word::Match), // match
"let" => Token::Word(Word::Let), // let
"import" => Token::Word(Word::Import), // import
"action" => Token::Word(Word::Action), // action
"struct" => Token::Word(Word::Struct), // struct
"enum" => Token::Word(Word::Enum), // enum
"effect" => Token::Word(Word::Effect), // trait
"impl" => Token::Word(Word::Impl), // impl
"when" => Token::Word(Word::When), // when
"use" => Token::Word(Word::Use), // use
"from" => Token::Word(Word::From), // from
"where" => Token::Word(Word::Where), // where
"self" => Token::Word(Word::Self_), // self
"for" => Token::Word(Word::For), // for
"pub" => Token::Word(Word::Pub), // pub
"priv" => Token::Word(Word::Priv), // priv
"#!" => Token::Shebang, // #!
"ident" => Token::Word(Word::Ident(<&'input str>)), // a-z, A-Z, 0-9, _
"string" => Token::String(<&'input str>), // "..."
// Comments
"comment" => Token::Comment(<&'input str>), // #
// Numbers
"int" => Token::Integer(<i64>), // 0-9
"float" => Token::Float(<f64>), // [0-9]*.0-9+
// Special
"eof" => Token::Eof, // EOF
"\n" => Token::NewLine, // \n
"(" => Token::LeftParen, // (
")" => Token::RightParen, // )
"{" => Token::LeftBrace, // {
"}" => Token::RightBrace, // }
"[" => Token::LeftBracket, // [
"]" => Token::RightBracket, // ]
"," => Token::Comma, // ,
":" => Token::Colon, // :
"." => Token::Dot, // .
"-" => Token::Minus, // -
"+" => Token::Plus, // +
"/" => Token::Divide, // /
"*" => Token::Multiply, // *
"%" => Token::Percent, // %
"$" => Token::Dollar, // $
"!" => Token::Exclamation, // !
"?" => Token::Question, // ?
"~" => Token::Tilde, // ~
"@" => Token::At, // @
"^" => Token::Caret, // ^
"->" => Token::Arrow, // ->
"=>" => Token::FatArrow, // =>
}
}
#[inline]
Span<T>: Spanned<T> = {
<@L> <T> <@R> => span!(<>)
};
#[inline]
Lines<T>: Vec<T> = {
<mut v:(<T> "\n")*> <e:T?> => match e {
None => v,
Some(e) => {
v.push(e);
v
}
}
}
#[inline]
Comma<T>: Vec<T> = { // (0)
<mut v:(<T> ",")*> <e:T?> => match e { // (1)
None=> v,
Some(e) => {
v.push(e);
v
}
}
};
#[inline]
Plus<T>: Vec<T> = {
<mut v:(<T> "+")*> <e:T?> => match e { // (1)
None=> v,
Some(e) => {
v.push(e);
v
}
}
};
#[inline]
Block<T>: Block<T> = {
"{" ("\n"?) <lines:Lines<T>> "}" => Block(lines)
};
// Keywords
None: Spanned<Keyword> = <lo:@L> "none" <hi:@R> => span!(lo, Keyword::None, hi);
Fn: Spanned<Keyword> = <lo:@L> "fn" <hi:@R> => span!(lo, Keyword::Fn, hi);
Effect: Spanned<Keyword> = <lo:@L> "effect" <hi:@R> => span!(lo, Keyword::Effect, hi);
Struct: Spanned<Keyword> = <lo:@L> "struct" <hi:@R> => span!(lo, Keyword::Struct, hi);
If: Spanned<Keyword> = <lo:@L> "if" <hi:@R> => span!(lo, Keyword::If, hi);
Else: Spanned<Keyword> = <lo:@L> "else" <hi:@R> => span!(lo, Keyword::Else, hi);
When: Spanned<Keyword> = <lo:@L> "when" <hi:@R> => span!(lo, Keyword::When, hi);
Use: Spanned<Keyword> = <lo:@L> "use" <hi:@R> => span!(lo, Keyword::Use, hi);
From: Spanned<Keyword> = <lo:@L> "from" <hi:@R> => span!(lo, Keyword::From, hi);
Impl: Spanned<Keyword> = <lo:@L> "impl" <hi:@R> => span!(lo, Keyword::Impl, hi);
Let: Spanned<Keyword> = <lo:@L> "let" <hi:@R> => span!(lo, Keyword::Let, hi);
True: Node = "true" => Node::Bool(true);
False: Node = "false" => Node::Bool(false);
KeywordAndVisibility<T>: Spanned<KeywordAndVisibility> = {
<lo:@L> <v:Visibility> <k:T> <hi:@R> => span!(lo, KeywordAndVisibility(k, v), hi),
};
Ident: Spanned<Ident> = {
<l:@L> <i:"ident"> <r:@R> => span!(l,Ident(i.to_string(), None),r),
};
IdentWithGenerics: Spanned<Ident> = {
<l:@L> <i:"ident"> "<" <g:Comma<Ident>> ">" <r:@R> => span!(l,Ident(i.to_string(), Some(g)),r),
};
IdentOrIdentWithGenerics: Spanned<Ident> = {
<i:Ident> => i,
<i:IdentWithGenerics> => i,
<l:@L> "self" <r:@R> => span!(l, Ident("self".to_string(), None),r),
};
Punctuated<T, Token>: Vec<T> = {
<mut v:(<T> <Token>)*> <e:T?> => match e {
None => v,
Some(e) => {
v.push(e);
v
}
}
};
Atom: Spanned<Value> = {
#[precedence(level="0")]
<l:@L> <i:"int"> <r:@R> => span!(l, Value::Literal(Literal::Integer(i)),r),
<l:@L> <f:"float"> <r:@R>=> span!(l, Value::Literal(Literal::Float(f)),r),
<l:@L> <s:"string"> <r:@R> => {
let start = 1;
let end = s.len() - 1;
span!(l, Value::Literal(Literal::String(s.get(start..end).expect(format!("malformed string {s}, strings must be quoted").as_str()).to_string())), r)
},
#[precedence(level="1")]
<i:Ident> => span!(i.0, Value::Ident(i.1), i.2),
};
String: Spanned<Node> = {
<l:@L> <s:"string"> <r:@R> => {
let start = 1;
let end = s.len() - 1;
span!(l, Node::String(s.get(start..end).expect(format!("malformed string {s}, strings must be quoted").as_str()).to_string()),r)
},
};
Expression: Spanned<Node> = {
#[precedence(level="1")]
Term,
#[precedence(level="2")] #[assoc(side="left")]
<lhs:Expression> "*" <rhs:Expression> => {
let (l, r) = (lhs.2, rhs.0);
span!(l,Node::BinaryExpression(BinaryOperation {
lhs: Box::new(lhs),
op: Operator::Mul,
rhs: Box::new(rhs)
}),r)
},
<l:@L> <lhs:Expression> "/" <rhs:Expression> <r:@R> => {
let (l, r) = (lhs.2, rhs.0);
span!(l,Node::BinaryExpression(BinaryOperation {
lhs: Box::new(lhs),
op: Operator::Div,
rhs: Box::new(rhs)
}),r)
},
#[precedence(level="3")] #[assoc(side="left")]
<lhs:Expression> "+" <rhs:Expression> => {
let (l, r) = (lhs.2, rhs.0);
span!(l,Node::BinaryExpression(BinaryOperation {
lhs: Box::new(lhs),
op: Operator::Add,
rhs: Box::new(rhs)
}),r)
},
<lhs:Expression> "-" <rhs:Expression> => {
let (l, r) = (lhs.2, rhs.0);
span!(l, Node::BinaryExpression(BinaryOperation {
lhs: Box::new(lhs),
op: Operator::Sub,
rhs: Box::new(rhs)
}),r)
},
};
FnCall: Spanned<Node> = {
<name:IdentOrIdentWithGenerics> "(" <args:Comma<Expression>> ")" <r:@R> => span!(name.0, Node::FnCall(FnCall(name, args)) ,r),
};
Term: Spanned<Node> = {
<s:Span<String>> => s.1,
<l:@L> <val:"int"> <r:@R> => span!(l, Node::Integer(val),r),
<i:Ident> => {
let (l, r) = (i.0, i.2);
span!(l, Node::Ident(i), r)},
<f:FnCall> => <>,
<l:@L> <true_:True> <r:@R> => span!(l,true_, r),
<l:@L> <false_:False> <r:@R> => span!(l,false_, r),
"(" <Expression> ")",
};
IdentOrIdentWithGenericsOrFnCall: Spanned<Node> = {
<i:IdentOrIdentWithGenerics> => {
let (l, r) = (i.0, i.2);
span!(l, Node::Ident(i), r)
},
<f:FnCall> => f,
};
FieldAccess: Spanned<Node> = {
<lhs:FieldAccess> "." <rhs:IdentOrIdentWithGenericsOrFnCall> => {
let (l, r) = (lhs.0, rhs.2);
span!(l,Node::FieldAccess(FieldAccess(Box::new(lhs), Box::new(rhs))),r)
},
<lhs:IdentOrIdentWithGenericsOrFnCall> => lhs,
};
Field: Spanned<FieldDef> = {
<vis:Visibility> <name:Ident> ":" <ty:IdentOrIdentWithGenerics> => {
let (l, r) = (name.0, ty.2);
span!(l, FieldDef(vis, name, ty), r)
}
};
TypeParameters: Spanned<Vec<Spanned<Ident>>> =
<l:@L> "<" <is:Comma<Ident>> ">" <r:@R> => span!(l,is ,r);
FnArg: Spanned<FnArg> = {
<self_:Span<"self">> => span!(self_.0, FnArg::Reciever, self_.2),
<field:Span<Field>> => {
let (l, r) = (field.0, field.2);
span!(l, FnArg::Field(field.1), r)
},
};
Prototype: Spanned<Prototype> = {
<l:@L> <name:IdentOrIdentWithGenerics> "("<args:Comma<FnArg>> ")" "[" <effects:Comma<Ident>> "]" <ret:("->" Ident)?> <r:@R> => {
let ret = match ret {
None => None,
Some(r) => Some(r.1),
};
span!(l, Prototype{name, args, ret, effects},r)
}
};
Statement: Spanned<Node> = {
<l:@L> Let <name:Ident> "=" <value:Expression> <r:@R> => span!(l, Node::Binding(Binding(name, Box::new(value))),r),
<IfDef> => <>,
FieldAccess => <>,
};
Visibility: Spanned<Visibility> = {
<l:@L> "pub" <r:@R> => span!(l, Visibility::Public, r),
<l:@L> "priv" <r:@R> => span!(l, Visibility::Private, r),
// elided
<l:@L> () <r:@R> => span!(l, Visibility::Private, r),
};
WhenBlock: (Spanned<Ident>,Block<Spanned<Node>>) = {
<l:@L> When <i:Ident> <lines:Block<Statement>> <r:@R> => (i, lines)
};
FnDef: Spanned<Node> = {
<l:@L> <kwv:KeywordAndVisibility<Fn>> <proto:Prototype> <block:Block<Statement>> <r:@R> => span!(l, Node::FnDef(FnDef(kwv,proto, block)), r),
};
EffectDef: Spanned<Node> = {
<l:@L> <kwv:KeywordAndVisibility<Effect>> <i:Ident> ":" <effects:Plus<Ident>> <block:Block<Prototype>> <r:@R> => span!(l,Node::EffectDef(EffectDef(kwv, i, effects,block)), r),
};
StructDef: Spanned<Node> = {
<l:@L> <kwv:KeywordAndVisibility<Struct>> <i:Ident> <fields:Block<Field>> <r:@R> => span!(l, Node::StructDef(StructDef(kwv, i, fields)),r),
};
IfDef: Spanned<Node> = {
<l:@L> If <cl:@L><cond:Statement><cr:@R> <if_:Block<Statement>> <r:@R> => {
let branch = BranchDef (
Box::new(cond),
vec![
(span!(l, Node::Bool(true), cl), if_),
]
);
span!(l, Node::Branch(branch), r)
},
<l:@L> If <cl:@L> <cond:Statement> <cr:@R> <if_:Block<Statement>> <el:@L> Else <er:@R> <else_:Block<Statement>> <r:@R> => {
let branch = BranchDef (
Box::new(cond),
vec![
(span!(l,Node::Bool(true),cl), if_),
(span!(el, Node::Bool(false), er), else_),
]
);
span!(l, Node::Branch(branch), r)
},
};
UseDef: Spanned<Node> = {
<l:@L> <kwv:KeywordAndVisibility<Use>> "{" <imports:Comma<Ident>> "}" From <i:Ident> <r:@R> => {
span!(l, Node::UseDef(UseDef(kwv,imports, i)), r)
},
};
ImplDef: Spanned<Node> = {
<l:@L> <kwv:KeywordAndVisibility<Impl>> <i:Ident> <t:("for" Ident)?> <lines:Block<FnDef>> <r:@R> => span!(l,Node::ImplDef(ImplDef(kwv, i, t.map(|t| t.1), lines)),r),
};
TopLevel: Spanned<Node> = {
<FnDef> => <>,
<EffectDef> => <>,
<StructDef> => <>,
<UseDef> => <>,
<ImplDef> => <>,
};
pub Source: Module = {
<expr:("\n"* TopLevel)*> => Module(expr.into_iter().map(|e| e.1).collect()),
! => {
errors.push(<>);
Module(vec![])
}
};
Examples
innitguv
use { native_fs, native_exec } from host
use { fs } from std
struct Innitguv {
fs: native_fs,
exec: native_exec
current_pid: i32
}
impl Exec for Innitguv {
fn exec(&self, arg0: str, args: vec<str>) [nd, exec, await] -> i32 {
let path = arg0
let pid = self.exec.exec(path, args)
if pid == -1 {
return -1
}
self.current_pid = pid
yield()
}
}
impl Actor for Innitguv {
fn recv(&self, msg: Message) [recv, await] {
self.exec(msg.path, msg.args)
}
}
Skills
Research
Crates
Crate srclang
src-lang
src-lang is a compiler is a language and a specification for a domain specific language that is aimed at manipulating source code.
Modules
analyzer
:analyzer
is responsible for analyzing the syntax tree and building the symbol table.ast
:ast
contains the abstract syntax tree for the src-lang.compiler
:compiler
contains the compiler for the src-lang.lexer
:lexer
contains the intermediate representation for the src-lang.ops
:ops
contains the operations tree traversal operations for the src-lang.parser
:parser
contains the parser for the src-lang, which is written in LALRPOP.
Macros
span
: A macro to create aSpanned<T>
object Heavily used in `src.lalrpop to create a Spanned object
Structs
Jar
: The salsa database for the compiler. The default convention is to implement this at the crate level.
Traits
Db
: The Db trait is a marker trait that is used to ensure that the Jar struct is a valid salsa database. The default convention is to implement this at the crate level.
Module srclang::analyzer
analyzer
is responsible for analyzing the syntax tree and building the symbol table.
Modules
db
:
Structs
SyntaxTree
:Url
:add_file
:get_symbol
:span_text
:
Functions
add_file
:get_symbol
:span_text
:
Module srclang::analyzer::db
Structs
Database
: The salsa database for the compiler.
Struct srclang::analyzer::db::Database
pub struct Database { /* private fields */ }
The salsa database for the compiler.
Trait Implementations
impl AsSalsaDatabase for Database
fn as_salsa_database(&self) -> &dyn Database
impl Database for Database
fn salsa_event(&self, event: Event)
This function is invoked at key points in the salsa runtime. It permits the database to be customized and to inject logging or other custom behavior. Read more
fn synthetic_write(&mut self, durability: Durability)
A “synthetic write” causes the system to act as though some input of durability durability
has changed. This is mostly useful for profiling scenarios. Read more
fn report_untracked_read(&self)
Reports that the query depends on some state unknown to salsa. Read more
impl DbWithJar<Jar> for Database
fn as_jar_db<'db>(&'db self) -> &'db <Jar as Jar<'db>>::DynDbwhere 'db: 'db,
impl Default for Database
fn default() -> Database
Returns the “default value” for a type. Read more
impl HasJar<Jar> for Database
fn jar(&self) -> (&Jar, &Runtime)
fn jar_mut(&mut self) -> (&mut Jar, &mut Runtime)
impl HasJars for Database
type Jars = (Jar,)
fn jars(&self) -> (&Self::Jars, &Runtime)
fn jars_mut(&mut self) -> (&mut Self::Jars, &mut Runtime)
Gets mutable access to the jars. This will trigger a new revision and it will also cancel any ongoing work in the current revision.
fn create_jars(routes: &mut Routes<Self>) -> Box<Self::Jars>
impl HasJarsDyn for Database
fn runtime(&self) -> &Runtime
fn runtime_mut(&mut self) -> &mut Runtime
fn maybe_changed_after( &self, input: DependencyIndex, revision: Revision) -> bool
fn cycle_recovery_strategy( &self, ingredient_index: IngredientIndex) -> CycleRecoveryStrategy
fn origin(&self, index: DatabaseKeyIndex) -> Option<QueryOrigin>
fn mark_validated_output( &self, executor: DatabaseKeyIndex, output: DependencyIndex)
fn remove_stale_output( &self, executor: DatabaseKeyIndex, stale_output: DependencyIndex)
Invoked when executor
used to output stale_output
but no longer does. This method routes that into a call to the remove_stale_output
method on the ingredient for stale_output
.
fn salsa_struct_deleted(&self, ingredient: IngredientIndex, id: Id)
Informs ingredient
that the salsa struct with id id
has been deleted. This means that id
will not be used in this revision and hence any memoized values keyed by that struct can be discarded. Read more
fn fmt_index(&self, index: DependencyIndex, fmt: &mut Formatter<'_>) -> Result
impl JarFromJars<Jar> for Database
fn jar_from_jars<'db>(jars: &Self::Jars) -> &Jar
fn jar_from_jars_mut<'db>(jars: &mut Self::Jars) -> &mut Jar
impl ParallelDatabase for Database
fn snapshot(&self) -> Snapshot<Self>
Creates a second handle to the database that holds the database fixed at a particular revision. So long as this “frozen” handle exists, any attempt to set
an input will block. Read more
Auto Trait Implementations
impl !Freeze for Database
impl !RefUnwindSafe for Database
impl Send for Database
impl !Sync for Database
impl Unpin for Database
impl !UnwindSafe for Database
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<DB> Db for DBwhere DB: DbWithJar<Jar> + ?Sized,
Function srclang::analyzer::add_file
pub fn add_file(db: &dyn Db, url: Url, text: String) -> SourceProgram
Function srclang::analyzer::get_symbol
pub fn get_symbol(
_db: &dyn Db,
_src: SourceProgram,
_pos: ByteOrLineColOrCoordInterned
) -> Option<Spanned<Node>>
Function srclang::analyzer::span_text
pub fn span_text(db: &dyn Db, span: Spanned) -> String
Struct srclang::analyzer::SyntaxTree
pub struct SyntaxTree(/* private fields */);
Implementations
impl SyntaxTree
pub fn new(__db: &<Jar as Jar<'_>>::DynDb, exprs: Vec<Spanned<Node>>) -> Self
Trait Implementations
impl AsId for SyntaxTree
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for SyntaxTree
fn clone(&self) -> SyntaxTree
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for SyntaxTree
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for SyntaxTree
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<SyntaxTree> for Jar
fn ingredient(&self) -> &<SyntaxTree as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <SyntaxTree as IngredientsFor>::Ingredients
impl Hash for SyntaxTree
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for SyntaxTree
type Jar = Jar
type Ingredients = (InputFieldIngredient<SyntaxTree, Vec<Spanned<Node>>>, InputIngredient<SyntaxTree>)
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for SyntaxTree
fn cmp(&self, other: &SyntaxTree) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for SyntaxTree
fn eq(&self, other: &SyntaxTree) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for SyntaxTree
fn partial_cmp(&self, other: &SyntaxTree) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for SyntaxTreewhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for SyntaxTree
impl Eq for SyntaxTree
impl StructuralPartialEq for SyntaxTree
Auto Trait Implementations
impl Freeze for SyntaxTree
impl RefUnwindSafe for SyntaxTree
impl Send for SyntaxTree
impl Sync for SyntaxTree
impl Unpin for SyntaxTree
impl UnwindSafe for SyntaxTree
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::analyzer::Url
pub struct Url(/* private fields */);
Implementations
impl Url
pub fn new(__db: &<Jar as Jar<'_>>::DynDb, url: String) -> Self
pub fn url<'db>(self, __db: &'db <Jar as Jar<'_>>::DynDb) -> String
Trait Implementations
impl AsId for Url
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Url
fn clone(&self) -> Url
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Url
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Url
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Url> for Jar
fn ingredient(&self) -> &<Url as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Url as IngredientsFor>::Ingredients
impl Hash for Url
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Url
type Jar = Jar
type Ingredients = (InputFieldIngredient<Url, String>, InputIngredient<Url>)
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for Url
fn cmp(&self, other: &Url) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Url
fn eq(&self, other: &Url) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Url
fn partial_cmp(&self, other: &Url) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Urlwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for Url
impl Eq for Url
impl StructuralPartialEq for Url
Auto Trait Implementations
impl Freeze for Url
impl RefUnwindSafe for Url
impl Send for Url
impl Sync for Url
impl Unpin for Url
impl UnwindSafe for Url
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::analyzer::add_file
pub struct add_file { /* private fields */ }
Implementations
impl add_file
pub fn get<'__db>( db: &'__db dyn Db, url: Url, text: String) -> &'__db SourceProgram
pub fn set(db: &mut dyn Db, url: Url, text: String, __value: SourceProgram)
pub fn accumulated<'__db, __A: Accumulator>( db: &'__db dyn Db, url: Url, text: String) -> Vec<<__A as Accumulator>::Data>where <Jar as Jar<'__db>>::DynDb: HasJar<<__A as Accumulator>::Jar>,
Trait Implementations
impl Configuration for add_file
type Jar = Jar
type SalsaStruct = Url
The “salsa struct type” that this function is associated with. This can be just salsa::Id
for functions that intern their arguments and are not clearly associated with any one salsa struct.
type Key = Id
What key is used to index the memo. Typically a salsa struct id, but if this memoized function has multiple arguments it will be a salsa::Id
that results from interning those arguments.
type Value = SourceProgram
The value computed by the function.
const CYCLE_STRATEGY: CycleRecoveryStrategy = salsa::cycle::CycleRecoveryStrategy::Panic
Determines whether this function can recover from being a participant in a cycle (and, if so, how).
fn should_backdate_value(v1: &Self::Value, v2: &Self::Value) -> bool
Invokes after a new result new_value`` has been computed for which an older memoized value existed
old_value`. Returns true if the new value is equal to the older one and hence should be “backdated” (i.e., marked as having last changed in an older revision, even though it was recomputed). Read more
fn execute(__db: &DynDb<'_, Self>, __id: Self::Key) -> Self::Value
Invoked when we need to compute the value for the given key, either because we’ve never computed it before or because the old one relied on inputs that have changed. Read more
fn recover_from_cycle( _db: &DynDb<'_, Self>, _cycle: &Cycle, _key: Self::Key) -> Self::Value
If the cycle strategy is Recover
, then invoked when key
is a participant in a cycle to find out what value it should have. Read more
fn key_from_id(id: Id) -> Self::Key
Given a salsa Id, returns the key. Convenience function to avoid having to type <C::Key as AsId>::from_id
.
impl HasIngredientsFor<add_file> for Jar
fn ingredient(&self) -> &<add_file as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <add_file as IngredientsFor>::Ingredients
impl IngredientsFor for add_file
type Ingredients = add_file
type Jar = Jar
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
Auto Trait Implementations
impl !Freeze for add_file
impl !RefUnwindSafe for add_file
impl Send for add_file
impl Sync for add_file
impl Unpin for add_file
impl UnwindSafe for add_file
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::analyzer::get_symbol
pub struct get_symbol { /* private fields */ }
Implementations
impl get_symbol
pub fn get<'__db>( _db: &'__db dyn Db, _src: SourceProgram, _pos: ByteOrLineColOrCoordInterned) -> &'__db Option<Spanned<Node>>
pub fn set( _db: &mut dyn Db, _src: SourceProgram, _pos: ByteOrLineColOrCoordInterned, __value: Option<Spanned<Node>> )
pub fn accumulated<'__db, __A: Accumulator>( _db: &'__db dyn Db, _src: SourceProgram, _pos: ByteOrLineColOrCoordInterned) -> Vec<<__A as Accumulator>::Data>where <Jar as Jar<'__db>>::DynDb: HasJar<<__A as Accumulator>::Jar>,
Trait Implementations
impl Configuration for get_symbol
type Jar = Jar
type SalsaStruct = SourceProgram
The “salsa struct type” that this function is associated with. This can be just salsa::Id
for functions that intern their arguments and are not clearly associated with any one salsa struct.
type Key = Id
What key is used to index the memo. Typically a salsa struct id, but if this memoized function has multiple arguments it will be a salsa::Id
that results from interning those arguments.
type Value = Option<Spanned<Node>>
The value computed by the function.
const CYCLE_STRATEGY: CycleRecoveryStrategy = salsa::cycle::CycleRecoveryStrategy::Panic
Determines whether this function can recover from being a participant in a cycle (and, if so, how).
fn should_backdate_value(v1: &Self::Value, v2: &Self::Value) -> bool
Invokes after a new result new_value`` has been computed for which an older memoized value existed
old_value`. Returns true if the new value is equal to the older one and hence should be “backdated” (i.e., marked as having last changed in an older revision, even though it was recomputed). Read more
fn execute(__db: &DynDb<'_, Self>, __id: Self::Key) -> Self::Value
Invoked when we need to compute the value for the given key, either because we’ve never computed it before or because the old one relied on inputs that have changed. Read more
fn recover_from_cycle( _db: &DynDb<'_, Self>, _cycle: &Cycle, _key: Self::Key) -> Self::Value
If the cycle strategy is Recover
, then invoked when key
is a participant in a cycle to find out what value it should have. Read more
fn key_from_id(id: Id) -> Self::Key
Given a salsa Id, returns the key. Convenience function to avoid having to type <C::Key as AsId>::from_id
.
impl HasIngredientsFor<get_symbol> for Jar
fn ingredient(&self) -> &<get_symbol as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <get_symbol as IngredientsFor>::Ingredients
impl IngredientsFor for get_symbol
type Ingredients = get_symbol
type Jar = Jar
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
Auto Trait Implementations
impl !Freeze for get_symbol
impl !RefUnwindSafe for get_symbol
impl Send for get_symbol
impl Sync for get_symbol
impl Unpin for get_symbol
impl UnwindSafe for get_symbol
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::analyzer::span_text
pub struct span_text { /* private fields */ }
Implementations
impl span_text
pub fn get<'__db>(db: &'__db dyn Db, span: Spanned) -> &'__db String
pub fn set(db: &mut dyn Db, span: Spanned, __value: String)
pub fn accumulated<'__db, __A: Accumulator>( db: &'__db dyn Db, span: Spanned) -> Vec<<__A as Accumulator>::Data>where <Jar as Jar<'__db>>::DynDb: HasJar<<__A as Accumulator>::Jar>,
Trait Implementations
impl Configuration for span_text
type Jar = Jar
type SalsaStruct = Spanned
The “salsa struct type” that this function is associated with. This can be just salsa::Id
for functions that intern their arguments and are not clearly associated with any one salsa struct.
type Key = Spanned
What key is used to index the memo. Typically a salsa struct id, but if this memoized function has multiple arguments it will be a salsa::Id
that results from interning those arguments.
type Value = String
The value computed by the function.
const CYCLE_STRATEGY: CycleRecoveryStrategy = salsa::cycle::CycleRecoveryStrategy::Panic
Determines whether this function can recover from being a participant in a cycle (and, if so, how).
fn should_backdate_value(v1: &Self::Value, v2: &Self::Value) -> bool
Invokes after a new result new_value`` has been computed for which an older memoized value existed
old_value`. Returns true if the new value is equal to the older one and hence should be “backdated” (i.e., marked as having last changed in an older revision, even though it was recomputed). Read more
fn execute(__db: &DynDb<'_, Self>, __id: Self::Key) -> Self::Value
Invoked when we need to compute the value for the given key, either because we’ve never computed it before or because the old one relied on inputs that have changed. Read more
fn recover_from_cycle( _db: &DynDb<'_, Self>, _cycle: &Cycle, _key: Self::Key) -> Self::Value
If the cycle strategy is Recover
, then invoked when key
is a participant in a cycle to find out what value it should have. Read more
fn key_from_id(id: Id) -> Self::Key
Given a salsa Id, returns the key. Convenience function to avoid having to type <C::Key as AsId>::from_id
.
impl HasIngredientsFor<span_text> for Jar
fn ingredient(&self) -> &<span_text as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <span_text as IngredientsFor>::Ingredients
impl IngredientsFor for span_text
type Ingredients = span_text
type Jar = Jar
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
Auto Trait Implementations
impl !Freeze for span_text
impl !RefUnwindSafe for span_text
impl Send for span_text
impl Sync for span_text
impl Unpin for span_text
impl UnwindSafe for span_text
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Module srclang::ast
ast
contains the abstract syntax tree for the src-lang.
Structs
Field
:Ident
:Keyword
:
Enums
Kw
:Literal
: An enum representing the different types of literals that can be used in an expression.Node
:Operator
: An enum representing the different operators that can be used in an expression.Visibility
: An enum representing the visibility of a field or method.
Traits
FieldVisitor
:IdentVisitor
:KeywordVisitor
:
Enum srclang::ast::Kw
pub enum Kw {
None,
Some,
Let,
Public,
Private,
Fn,
If,
Else,
Match,
Arrow,
Struct,
SelfValue,
Effect,
Impl,
Use,
}
Variants
None
The None
keyword.
Some
The Some
keyword.
Let
The let
keyword.
Public
The pub
keyword.
Private
The priv
keyword.
Fn
The fn
keyword.
If
The if
keyword.
Else
The else
keyword.
Match
The match
keyword.
Arrow
The while
keyword.
Struct
The struct
keyword.
SelfValue
The self
keyword.
Effect
The effect
keyword.
Impl
The impl
keyword.
Use
The use
keyword.
Trait Implementations
impl Debug for Kw
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
Auto Trait Implementations
impl Freeze for Kw
impl RefUnwindSafe for Kw
impl Send for Kw
impl Sync for Kw
impl Unpin for Kw
impl UnwindSafe for Kw
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::ast::Literal
pub enum Literal {
Bool(bool),
Float(f64),
Integer(i64),
String(String),
}
An enum representing the different types of literals that can be used in an expression.
Variants
Bool(bool)
Float(f64)
Integer(i64)
String(String)
Auto Trait Implementations
impl Freeze for Literal
impl RefUnwindSafe for Literal
impl Send for Literal
impl Sync for Literal
impl Unpin for Literal
impl UnwindSafe for Literal
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::ast::Node
pub enum Node {
Ident(Ident),
Field(Field),
Literal(Literal),
Visibility(Visibility),
Operator(Operator),
}
Variants
Ident(Ident)
Field(Field)
Literal(Literal)
Visibility(Visibility)
Operator(Operator)
Auto Trait Implementations
impl Freeze for Node
impl RefUnwindSafe for Node
impl Send for Node
impl Sync for Node
impl Unpin for Node
impl UnwindSafe for Node
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::ast::Operator
pub enum Operator {
Add,
Sub,
Mul,
Div,
Mod,
And,
Or,
Not,
Eq,
Ne,
Lt,
Le,
Gt,
Ge,
}
An enum representing the different operators that can be used in an expression.
Variants
Add
Sub
Mul
Div
Mod
And
Or
Not
Eq
Ne
Lt
Le
Gt
Ge
Auto Trait Implementations
impl Freeze for Operator
impl RefUnwindSafe for Operator
impl Send for Operator
impl Sync for Operator
impl Unpin for Operator
impl UnwindSafe for Operator
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::ast::Visibility
pub enum Visibility {
Private,
Public,
}
An enum representing the visibility of a field or method.
Variants
Private
Public
Trait Implementations
impl Debug for Visibility
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for Visibility
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
Auto Trait Implementations
impl Freeze for Visibility
impl RefUnwindSafe for Visibility
impl Send for Visibility
impl Sync for Visibility
impl Unpin for Visibility
impl UnwindSafe for Visibility
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::ast::Field
pub struct Field { /* private fields */ }
Trait Implementations
impl Debug for Field
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl From<(Option<Spanned<Visibility>>, Spanned<String>, Spanned<Ident>)> for Field
fn from( field: (Option<Spanned<Visibility>>, Spanned<String>, Spanned<Ident>) ) -> Self
Converts to this type from the input type.
Auto Trait Implementations
impl Freeze for Field
impl RefUnwindSafe for Field
impl Send for Field
impl Sync for Field
impl Unpin for Field
impl UnwindSafe for Field
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::ast::Ident
pub struct Ident { /* private fields */ }
Trait Implementations
impl Debug for Ident
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl From<(Spanned<String>, Vec<Spanned<Ident>>)> for Ident
fn from(ident: (Spanned<String>, Vec<Spanned<Ident>>)) -> Self
Converts to this type from the input type.
Auto Trait Implementations
impl Freeze for Ident
impl RefUnwindSafe for Ident
impl Send for Ident
impl Sync for Ident
impl Unpin for Ident
impl UnwindSafe for Ident
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::ast::Keyword
pub struct Keyword { /* private fields */ }
Trait Implementations
impl Debug for Keyword
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl From<Spanned<Kw>> for Keyword
fn from(keyword: Spanned<Kw>) -> Self
Converts to this type from the input type.
Auto Trait Implementations
impl Freeze for Keyword
impl RefUnwindSafe for Keyword
impl Send for Keyword
impl Sync for Keyword
impl Unpin for Keyword
impl UnwindSafe for Keyword
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Trait srclang::ast::FieldVisitor
pub trait FieldVisitor {
// Required methods
fn visit_vis(&self, node: &Visibility, span: &Range<Location>) -> Control;
fn visit_name(&self, node: &String, span: &Range<Location>) -> Control;
fn visit_ty(&self, node: &Ident, span: &Range<Location>) -> Control;
}
Required Methods
fn visit_vis(&self, node: &Visibility, span: &Range<Location>) -> Control
fn visit_name(&self, node: &String, span: &Range<Location>) -> Control
fn visit_ty(&self, node: &Ident, span: &Range<Location>) -> Control
Implementors
Trait srclang::ast::IdentVisitor
pub trait IdentVisitor {
// Required methods
fn visit_name(&self, node: &String, span: &Range<Location>) -> Control;
fn visit_generics(&self, node: &Ident, span: &Range<Location>) -> Control;
}
Required Methods
fn visit_name(&self, node: &String, span: &Range<Location>) -> Control
fn visit_generics(&self, node: &Ident, span: &Range<Location>) -> Control
Implementors
Trait srclang::ast::KeywordVisitor
pub trait KeywordVisitor {
// Required method
fn visit_kw(&self, node: &Kw, span: &Range<Location>) -> Control;
}
Required Methods
fn visit_kw(&self, node: &Kw, span: &Range<Location>) -> Control
Implementors
Module srclang::compiler
compiler
contains the compiler for the src-lang.
Modules
errors
:ir
:text
:
Structs
add_imports
:compile
:compile_effect
:
Functions
add_imports
:compile
:compile_effect
:
Module srclang::compiler::errors
Structs
Errors
:
Struct srclang::compiler::errors::Errors
pub struct Errors<'a>(/* private fields */);
Trait Implementations
impl<'a> From<Vec<ErrorRecovery<Location, Token<'a>, &'a str>>> for Errors<'a>
fn from(value: Vec<ErrorRecovery<Location, Token<'a>, &'a str>>) -> Self
Converts to this type from the input type.
impl<'a> IntoIterator for Errors<'a>
type Item = Range<Location>
The type of the elements being iterated over.
type IntoIter = <Vec<Range<Location>> as IntoIterator>::IntoIter
Which kind of iterator are we turning this into?
fn into_iter(self) -> Self::IntoIter
Creates an iterator from a value. Read more
Auto Trait Implementations
impl<'a> Freeze for Errors<'a>
impl<'a> RefUnwindSafe for Errors<'a>
impl<'a> Send for Errors<'a>
impl<'a> Sync for Errors<'a>
impl<'a> Unpin for Errors<'a>
impl<'a> UnwindSafe for Errors<'a>
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Function srclang::compiler::add_imports
pub fn add_imports(db: &dyn Db, import: Import) -> Vec<Mangled>
Function srclang::compiler::compile
pub fn compile(db: &dyn Db, src: SourceProgram) -> Program
Function srclang::compiler::compile_effect
pub fn compile_effect(_db: &dyn Db, _effect: EffectDef)
Module srclang::compiler::ir
Structs
EffectDef
:Function
:Import
:InternedEffect
:Mangled
:Program
:Symbol
:__EffectDefConfig
:__FunctionConfig
:__ImportConfig
:__InternedEffectData
: Internal struct used for interned item__MangledData
: Internal struct used for interned item__ProgramConfig
:__SymbolData
: Internal struct used for interned item
Struct srclang::compiler::ir::EffectDef
pub struct EffectDef(/* private fields */);
Implementations
impl EffectDef
pub fn new(__db: &<Jar as Jar<'_>>::DynDb, effect: EffectDef) -> Self
pub fn effect<'db>(self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db EffectDef
Trait Implementations
impl AsId for EffectDef
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for EffectDef
fn clone(&self) -> EffectDef
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for EffectDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for EffectDef
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<EffectDef> for Jar
fn ingredient(&self) -> &<EffectDef as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <EffectDef as IngredientsFor>::Ingredients
impl Hash for EffectDef
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for EffectDef
type Jar = Jar
type Ingredients = (TrackedStructIngredient<__EffectDefConfig>, [TrackedFieldIngredient<__EffectDefConfig>; 1])
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for EffectDef
fn cmp(&self, other: &EffectDef) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for EffectDef
fn eq(&self, other: &EffectDef) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for EffectDef
fn partial_cmp(&self, other: &EffectDef) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for EffectDefwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(db: &DB, index: IngredientIndex)
impl<DB> TrackedStructInDb<DB> for EffectDefwhere DB: ?Sized + DbWithJar<Jar>,
fn database_key_index(self, db: &DB) -> DatabaseKeyIndex
Converts the identifier for this tracked struct into a DatabaseKeyIndex
.
impl Copy for EffectDef
impl Eq for EffectDef
impl StructuralPartialEq for EffectDef
Auto Trait Implementations
impl Freeze for EffectDef
impl RefUnwindSafe for EffectDef
impl Send for EffectDef
impl Sync for EffectDef
impl Unpin for EffectDef
impl UnwindSafe for EffectDef
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::ir::Function
pub struct Function(/* private fields */);
Implementations
impl Function
pub fn new( __db: &<Jar as Jar<'_>>::DynDb, name: String, body: Vec<Box<Function>>, effects: Vec<InternedEffect> ) -> Self
pub fn name<'db>(self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db String
pub fn body<'db>( self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db Vec<Box<Function>>
pub fn effects<'db>( self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db Vec<InternedEffect>
Trait Implementations
impl AsId for Function
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Function
fn clone(&self) -> Function
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Function
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Function
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Function> for Jar
fn ingredient(&self) -> &<Function as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Function as IngredientsFor>::Ingredients
impl Hash for Function
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Function
type Jar = Jar
type Ingredients = (TrackedStructIngredient<__FunctionConfig>, [TrackedFieldIngredient<__FunctionConfig>; 3])
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for Function
fn cmp(&self, other: &Function) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Function
fn eq(&self, other: &Function) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Function
fn partial_cmp(&self, other: &Function) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Functionwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(db: &DB, index: IngredientIndex)
impl<DB> TrackedStructInDb<DB> for Functionwhere DB: ?Sized + DbWithJar<Jar>,
fn database_key_index(self, db: &DB) -> DatabaseKeyIndex
Converts the identifier for this tracked struct into a DatabaseKeyIndex
.
impl Copy for Function
impl Eq for Function
impl StructuralPartialEq for Function
Auto Trait Implementations
impl Freeze for Function
impl RefUnwindSafe for Function
impl Send for Function
impl Sync for Function
impl Unpin for Function
impl UnwindSafe for Function
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::ir::Import
pub struct Import(/* private fields */);
Implementations
impl Import
pub fn new( __db: &<Jar as Jar<'_>>::DynDb, imports: Vec<String>, module: String) -> Self
pub fn imports<'db>( self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db Vec<String>
pub fn module<'db>(self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db String
Trait Implementations
impl AsId for Import
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Import
fn clone(&self) -> Import
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Import
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Import
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Import> for Jar
fn ingredient(&self) -> &<Import as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Import as IngredientsFor>::Ingredients
impl Hash for Import
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Import
type Jar = Jar
type Ingredients = (TrackedStructIngredient<__ImportConfig>, [TrackedFieldIngredient<__ImportConfig>; 2])
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for Import
fn cmp(&self, other: &Import) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Import
fn eq(&self, other: &Import) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Import
fn partial_cmp(&self, other: &Import) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Importwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(db: &DB, index: IngredientIndex)
impl<DB> TrackedStructInDb<DB> for Importwhere DB: ?Sized + DbWithJar<Jar>,
fn database_key_index(self, db: &DB) -> DatabaseKeyIndex
Converts the identifier for this tracked struct into a DatabaseKeyIndex
.
impl Copy for Import
impl Eq for Import
impl StructuralPartialEq for Import
Auto Trait Implementations
impl Freeze for Import
impl RefUnwindSafe for Import
impl Send for Import
impl Sync for Import
impl Unpin for Import
impl UnwindSafe for Import
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::ir::InternedEffect
pub struct InternedEffect(/* private fields */);
Implementations
impl InternedEffect
pub fn effect(self, db: &<Jar as Jar<'_>>::DynDb) -> String
pub fn new(db: &<Jar as Jar<'_>>::DynDb, effect: String) -> Self
Trait Implementations
impl AsId for InternedEffect
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for InternedEffect
fn clone(&self) -> InternedEffect
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for InternedEffect
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for InternedEffect
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<InternedEffect> for Jar
fn ingredient(&self) -> &<InternedEffect as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <InternedEffect as IngredientsFor>::Ingredients
impl Hash for InternedEffect
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for InternedEffect
type Jar = Jar
type Ingredients = InternedIngredient<InternedEffect, __InternedEffectData>
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: JarFromJars<Self::Jar>,
impl Ord for InternedEffect
fn cmp(&self, other: &InternedEffect) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for InternedEffect
fn eq(&self, other: &InternedEffect) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for InternedEffect
fn partial_cmp(&self, other: &InternedEffect) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for InternedEffectwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for InternedEffect
impl Eq for InternedEffect
impl StructuralPartialEq for InternedEffect
Auto Trait Implementations
impl Freeze for InternedEffect
impl RefUnwindSafe for InternedEffect
impl Send for InternedEffect
impl Sync for InternedEffect
impl Unpin for InternedEffect
impl UnwindSafe for InternedEffect
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::ir::Mangled
pub struct Mangled(/* private fields */);
Implementations
impl Mangled
pub fn mangled<'db>(self, db: &'db <Jar as Jar<'_>>::DynDb) -> &'db String
pub fn new(db: &<Jar as Jar<'_>>::DynDb, mangled: String) -> Self
Trait Implementations
impl AsId for Mangled
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Mangled
fn clone(&self) -> Mangled
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Mangled
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Mangled
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Mangled> for Jar
fn ingredient(&self) -> &<Mangled as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Mangled as IngredientsFor>::Ingredients
impl Hash for Mangled
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Mangled
type Jar = Jar
type Ingredients = InternedIngredient<Mangled, __MangledData>
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: JarFromJars<Self::Jar>,
impl Ord for Mangled
fn cmp(&self, other: &Mangled) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Mangled
fn eq(&self, other: &Mangled) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Mangled
fn partial_cmp(&self, other: &Mangled) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Mangledwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for Mangled
impl Eq for Mangled
impl StructuralPartialEq for Mangled
Auto Trait Implementations
impl Freeze for Mangled
impl RefUnwindSafe for Mangled
impl Send for Mangled
impl Sync for Mangled
impl Unpin for Mangled
impl UnwindSafe for Mangled
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::ir::Program
pub struct Program(/* private fields */);
Implementations
impl Program
pub fn new( __db: &<Jar as Jar<'_>>::DynDb, modul: Vec<Function>, symbols: BTreeMap<Mangled, Symbol> ) -> Self
pub fn modul<'db>( self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db Vec<Function>
pub fn symbols<'db>( self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db BTreeMap<Mangled, Symbol>
Trait Implementations
impl AsId for Program
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Program
fn clone(&self) -> Program
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Program
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Program
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Program> for Jar
fn ingredient(&self) -> &<Program as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Program as IngredientsFor>::Ingredients
impl Hash for Program
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Program
type Jar = Jar
type Ingredients = (TrackedStructIngredient<__ProgramConfig>, [TrackedFieldIngredient<__ProgramConfig>; 2])
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for Program
fn cmp(&self, other: &Program) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Program
fn eq(&self, other: &Program) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Program
fn partial_cmp(&self, other: &Program) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Programwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(db: &DB, index: IngredientIndex)
impl<DB> TrackedStructInDb<DB> for Programwhere DB: ?Sized + DbWithJar<Jar>,
fn database_key_index(self, db: &DB) -> DatabaseKeyIndex
Converts the identifier for this tracked struct into a DatabaseKeyIndex
.
impl Copy for Program
impl Eq for Program
impl StructuralPartialEq for Program
Auto Trait Implementations
impl Freeze for Program
impl RefUnwindSafe for Program
impl Send for Program
impl Sync for Program
impl Unpin for Program
impl UnwindSafe for Program
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::ir::Symbol
pub struct Symbol(/* private fields */);
Implementations
impl Symbol
pub fn symbol<'db>(self, db: &'db <Jar as Jar<'_>>::DynDb) -> &'db Mangled
pub fn new(db: &<Jar as Jar<'_>>::DynDb, symbol: Mangled) -> Self
Trait Implementations
impl AsId for Symbol
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Symbol
fn clone(&self) -> Symbol
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Symbol
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Symbol
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Symbol> for Jar
fn ingredient(&self) -> &<Symbol as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Symbol as IngredientsFor>::Ingredients
impl Hash for Symbol
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Symbol
type Jar = Jar
type Ingredients = InternedIngredient<Symbol, __SymbolData>
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: JarFromJars<Self::Jar>,
impl Ord for Symbol
fn cmp(&self, other: &Symbol) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Symbol
fn eq(&self, other: &Symbol) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Symbol
fn partial_cmp(&self, other: &Symbol) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Symbolwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for Symbol
impl Eq for Symbol
impl StructuralPartialEq for Symbol
Auto Trait Implementations
impl Freeze for Symbol
impl RefUnwindSafe for Symbol
impl Send for Symbol
impl Sync for Symbol
impl Unpin for Symbol
impl UnwindSafe for Symbol
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::ir::__EffectDefConfig
pub struct __EffectDefConfig { /* private fields */ }
Trait Implementations
impl Configuration for __EffectDefConfig
type Id = EffectDef
The id type used to define instances of this struct. The TrackedStructIngredient
contains the interner that will create the id values.
type Fields = (EffectDef,)
A (possibly empty) tuple of the fields for this struct.
type Revisions = [Revision; 1]
A array of Revision
values, one per each of the value fields. When a struct is re-recreated in a new revision, the corresponding entries for each field are updated to the new revision if their values have changed (or if the field is marked as #[no_eq]
).
fn id_fields(fields: &Self::Fields) -> impl Hash
fn revision(revisions: &Self::Revisions, field_index: u32) -> Revision
Access the revision of a given value field.field_index
will be between 0 and the number of value fields.
fn new_revisions(current_revision: Revision) -> Self::Revisions
Create a new value revision array where each element is set to current_revision
.
fn update_revisions( current_revision_: Revision, old_value_: &Self::Fields, new_value_: &Self::Fields, revisions_: &mut Self::Revisions)
Update an existing value revision array revisions
, given the tuple of the old values (old_value
) and the tuple of the values (new_value
). If a value has changed, then its element is updated to current_revision
.
Auto Trait Implementations
impl Freeze for __EffectDefConfig
impl RefUnwindSafe for __EffectDefConfig
impl Send for __EffectDefConfig
impl Sync for __EffectDefConfig
impl Unpin for __EffectDefConfig
impl UnwindSafe for __EffectDefConfig
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::compiler::ir::__FunctionConfig
pub struct __FunctionConfig { /* private fields */ }
Trait Implementations
impl Configuration for __FunctionConfig
type Id = Function
The id type used to define instances of this struct. The TrackedStructIngredient
contains the interner that will create the id values.
type Fields = (String, Vec<Box<Function>>, Vec<InternedEffect>)
A (possibly empty) tuple of the fields for this struct.
type Revisions = [Revision; 3]
A array of Revision
values, one per each of the value fields. When a struct is re-recreated in a new revision, the corresponding entries for each field are updated to the new revision if their values have changed (or if the field is marked as #[no_eq]
).
fn id_fields(fields: &Self::Fields) -> impl Hash
fn revision(revisions: &Self::Revisions, field_index: u32) -> Revision
Access the revision of a given value field.field_index
will be between 0 and the number of value fields.
fn new_revisions(current_revision: Revision) -> Self::Revisions
Create a new value revision array where each element is set to current_revision
.
fn update_revisions( current_revision_: Revision, old_value_: &Self::Fields, new_value_: &Self::Fields, revisions_: &mut Self::Revisions)
Update an existing value revision array revisions
, given the tuple of the old values (old_value
) and the tuple of the values (new_value
). If a value has changed, then its element is updated to current_revision
.
Auto Trait Implementations
impl Freeze for __FunctionConfig
impl RefUnwindSafe for __FunctionConfig
impl Send for __FunctionConfig
impl Sync for __FunctionConfig
impl Unpin for __FunctionConfig
impl UnwindSafe for __FunctionConfig
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::compiler::ir::__ImportConfig
pub struct __ImportConfig { /* private fields */ }
Trait Implementations
impl Configuration for __ImportConfig
type Id = Import
The id type used to define instances of this struct. The TrackedStructIngredient
contains the interner that will create the id values.
type Fields = (Vec<String>, String)
A (possibly empty) tuple of the fields for this struct.
type Revisions = [Revision; 2]
A array of Revision
values, one per each of the value fields. When a struct is re-recreated in a new revision, the corresponding entries for each field are updated to the new revision if their values have changed (or if the field is marked as #[no_eq]
).
fn id_fields(fields: &Self::Fields) -> impl Hash
fn revision(revisions: &Self::Revisions, field_index: u32) -> Revision
Access the revision of a given value field.field_index
will be between 0 and the number of value fields.
fn new_revisions(current_revision: Revision) -> Self::Revisions
Create a new value revision array where each element is set to current_revision
.
fn update_revisions( current_revision_: Revision, old_value_: &Self::Fields, new_value_: &Self::Fields, revisions_: &mut Self::Revisions)
Update an existing value revision array revisions
, given the tuple of the old values (old_value
) and the tuple of the values (new_value
). If a value has changed, then its element is updated to current_revision
.
Auto Trait Implementations
impl Freeze for __ImportConfig
impl RefUnwindSafe for __ImportConfig
impl Send for __ImportConfig
impl Sync for __ImportConfig
impl Unpin for __ImportConfig
impl UnwindSafe for __ImportConfig
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::compiler::ir::__InternedEffectData
pub struct __InternedEffectData { /* private fields */ }
Internal struct used for interned item
Trait Implementations
impl Clone for __InternedEffectData
fn clone(&self) -> __InternedEffectData
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Hash for __InternedEffectData
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl PartialEq for __InternedEffectData
fn eq(&self, other: &__InternedEffectData) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Eq for __InternedEffectData
impl StructuralPartialEq for __InternedEffectData
Auto Trait Implementations
impl Freeze for __InternedEffectData
impl RefUnwindSafe for __InternedEffectData
impl Send for __InternedEffectData
impl Sync for __InternedEffectData
impl Unpin for __InternedEffectData
impl UnwindSafe for __InternedEffectData
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
Struct srclang::compiler::ir::__MangledData
pub struct __MangledData { /* private fields */ }
Internal struct used for interned item
Trait Implementations
impl Clone for __MangledData
fn clone(&self) -> __MangledData
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Hash for __MangledData
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl PartialEq for __MangledData
fn eq(&self, other: &__MangledData) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Eq for __MangledData
impl StructuralPartialEq for __MangledData
Auto Trait Implementations
impl Freeze for __MangledData
impl RefUnwindSafe for __MangledData
impl Send for __MangledData
impl Sync for __MangledData
impl Unpin for __MangledData
impl UnwindSafe for __MangledData
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
Struct srclang::compiler::ir::__ProgramConfig
pub struct __ProgramConfig { /* private fields */ }
Trait Implementations
impl Configuration for __ProgramConfig
type Id = Program
The id type used to define instances of this struct. The TrackedStructIngredient
contains the interner that will create the id values.
type Fields = (Vec<Function>, BTreeMap<Mangled, Symbol>)
A (possibly empty) tuple of the fields for this struct.
type Revisions = [Revision; 2]
A array of Revision
values, one per each of the value fields. When a struct is re-recreated in a new revision, the corresponding entries for each field are updated to the new revision if their values have changed (or if the field is marked as #[no_eq]
).
fn id_fields(fields: &Self::Fields) -> impl Hash
fn revision(revisions: &Self::Revisions, field_index: u32) -> Revision
Access the revision of a given value field.field_index
will be between 0 and the number of value fields.
fn new_revisions(current_revision: Revision) -> Self::Revisions
Create a new value revision array where each element is set to current_revision
.
fn update_revisions( current_revision_: Revision, old_value_: &Self::Fields, new_value_: &Self::Fields, revisions_: &mut Self::Revisions)
Update an existing value revision array revisions
, given the tuple of the old values (old_value
) and the tuple of the values (new_value
). If a value has changed, then its element is updated to current_revision
.
Auto Trait Implementations
impl Freeze for __ProgramConfig
impl RefUnwindSafe for __ProgramConfig
impl Send for __ProgramConfig
impl Sync for __ProgramConfig
impl Unpin for __ProgramConfig
impl UnwindSafe for __ProgramConfig
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::compiler::ir::__SymbolData
pub struct __SymbolData { /* private fields */ }
Internal struct used for interned item
Trait Implementations
impl Clone for __SymbolData
fn clone(&self) -> __SymbolData
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Hash for __SymbolData
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl PartialEq for __SymbolData
fn eq(&self, other: &__SymbolData) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Eq for __SymbolData
impl StructuralPartialEq for __SymbolData
Auto Trait Implementations
impl Freeze for __SymbolData
impl RefUnwindSafe for __SymbolData
impl Send for __SymbolData
impl Sync for __SymbolData
impl Unpin for __SymbolData
impl UnwindSafe for __SymbolData
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
Struct srclang::compiler::add_imports
pub struct add_imports { /* private fields */ }
Implementations
impl add_imports
pub fn get<'__db>(db: &'__db dyn Db, import: Import) -> &'__db Vec<Mangled>
pub fn set(db: &mut dyn Db, import: Import, __value: Vec<Mangled>)
pub fn accumulated<'__db, __A: Accumulator>( db: &'__db dyn Db, import: Import) -> Vec<<__A as Accumulator>::Data>where <Jar as Jar<'__db>>::DynDb: HasJar<<__A as Accumulator>::Jar>,
Trait Implementations
impl Configuration for add_imports
type Jar = Jar
type SalsaStruct = Import
The “salsa struct type” that this function is associated with. This can be just salsa::Id
for functions that intern their arguments and are not clearly associated with any one salsa struct.
type Key = Import
What key is used to index the memo. Typically a salsa struct id, but if this memoized function has multiple arguments it will be a salsa::Id
that results from interning those arguments.
type Value = Vec<Mangled>
The value computed by the function.
const CYCLE_STRATEGY: CycleRecoveryStrategy = salsa::cycle::CycleRecoveryStrategy::Panic
Determines whether this function can recover from being a participant in a cycle (and, if so, how).
fn should_backdate_value(v1: &Self::Value, v2: &Self::Value) -> bool
Invokes after a new result new_value`` has been computed for which an older memoized value existed
old_value`. Returns true if the new value is equal to the older one and hence should be “backdated” (i.e., marked as having last changed in an older revision, even though it was recomputed). Read more
fn execute(__db: &DynDb<'_, Self>, __id: Self::Key) -> Self::Value
Invoked when we need to compute the value for the given key, either because we’ve never computed it before or because the old one relied on inputs that have changed. Read more
fn recover_from_cycle( _db: &DynDb<'_, Self>, _cycle: &Cycle, _key: Self::Key) -> Self::Value
If the cycle strategy is Recover
, then invoked when key
is a participant in a cycle to find out what value it should have. Read more
fn key_from_id(id: Id) -> Self::Key
Given a salsa Id, returns the key. Convenience function to avoid having to type <C::Key as AsId>::from_id
.
impl HasIngredientsFor<add_imports> for Jar
fn ingredient(&self) -> &<add_imports as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <add_imports as IngredientsFor>::Ingredients
impl IngredientsFor for add_imports
type Ingredients = add_imports
type Jar = Jar
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
Auto Trait Implementations
impl !Freeze for add_imports
impl !RefUnwindSafe for add_imports
impl Send for add_imports
impl Sync for add_imports
impl Unpin for add_imports
impl UnwindSafe for add_imports
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::compiler::compile
pub struct compile { /* private fields */ }
Implementations
impl compile
pub fn get<'__db>(db: &'__db dyn Db, src: SourceProgram) -> &'__db Program
pub fn set(db: &mut dyn Db, src: SourceProgram, __value: Program)
pub fn accumulated<'__db, __A: Accumulator>( db: &'__db dyn Db, src: SourceProgram) -> Vec<<__A as Accumulator>::Data>where <Jar as Jar<'__db>>::DynDb: HasJar<<__A as Accumulator>::Jar>,
Trait Implementations
impl Configuration for compile
type Jar = Jar
type SalsaStruct = SourceProgram
The “salsa struct type” that this function is associated with. This can be just salsa::Id
for functions that intern their arguments and are not clearly associated with any one salsa struct.
type Key = SourceProgram
What key is used to index the memo. Typically a salsa struct id, but if this memoized function has multiple arguments it will be a salsa::Id
that results from interning those arguments.
type Value = Program
The value computed by the function.
const CYCLE_STRATEGY: CycleRecoveryStrategy = salsa::cycle::CycleRecoveryStrategy::Panic
Determines whether this function can recover from being a participant in a cycle (and, if so, how).
fn should_backdate_value(v1: &Self::Value, v2: &Self::Value) -> bool
Invokes after a new result new_value`` has been computed for which an older memoized value existed
old_value`. Returns true if the new value is equal to the older one and hence should be “backdated” (i.e., marked as having last changed in an older revision, even though it was recomputed). Read more
fn execute(__db: &DynDb<'_, Self>, __id: Self::Key) -> Self::Value
Invoked when we need to compute the value for the given key, either because we’ve never computed it before or because the old one relied on inputs that have changed. Read more
fn recover_from_cycle( _db: &DynDb<'_, Self>, _cycle: &Cycle, _key: Self::Key) -> Self::Value
If the cycle strategy is Recover
, then invoked when key
is a participant in a cycle to find out what value it should have. Read more
fn key_from_id(id: Id) -> Self::Key
Given a salsa Id, returns the key. Convenience function to avoid having to type <C::Key as AsId>::from_id
.
impl HasIngredientsFor<compile> for Jar
fn ingredient(&self) -> &<compile as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <compile as IngredientsFor>::Ingredients
impl IngredientsFor for compile
type Ingredients = compile
type Jar = Jar
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
Auto Trait Implementations
impl !Freeze for compile
impl !RefUnwindSafe for compile
impl Send for compile
impl Sync for compile
impl Unpin for compile
impl UnwindSafe for compile
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::compiler::compile_effect
pub struct compile_effect { /* private fields */ }
Implementations
impl compile_effect
pub fn get<'__db>(_db: &'__db dyn Db, _effect: EffectDef) -> &'__db ()
pub fn set(_db: &mut dyn Db, _effect: EffectDef, __value: ())
pub fn accumulated<'__db, __A: Accumulator>( _db: &'__db dyn Db, _effect: EffectDef) -> Vec<<__A as Accumulator>::Data>where <Jar as Jar<'__db>>::DynDb: HasJar<<__A as Accumulator>::Jar>,
Trait Implementations
impl Configuration for compile_effect
type Jar = Jar
type SalsaStruct = EffectDef
The “salsa struct type” that this function is associated with. This can be just salsa::Id
for functions that intern their arguments and are not clearly associated with any one salsa struct.
type Key = EffectDef
What key is used to index the memo. Typically a salsa struct id, but if this memoized function has multiple arguments it will be a salsa::Id
that results from interning those arguments.
type Value = ()
The value computed by the function.
const CYCLE_STRATEGY: CycleRecoveryStrategy = salsa::cycle::CycleRecoveryStrategy::Panic
Determines whether this function can recover from being a participant in a cycle (and, if so, how).
fn should_backdate_value(v1: &Self::Value, v2: &Self::Value) -> bool
Invokes after a new result new_value`` has been computed for which an older memoized value existed
old_value`. Returns true if the new value is equal to the older one and hence should be “backdated” (i.e., marked as having last changed in an older revision, even though it was recomputed). Read more
fn execute(__db: &DynDb<'_, Self>, __id: Self::Key) -> Self::Value
Invoked when we need to compute the value for the given key, either because we’ve never computed it before or because the old one relied on inputs that have changed. Read more
fn recover_from_cycle( _db: &DynDb<'_, Self>, _cycle: &Cycle, _key: Self::Key) -> Self::Value
If the cycle strategy is Recover
, then invoked when key
is a participant in a cycle to find out what value it should have. Read more
fn key_from_id(id: Id) -> Self::Key
Given a salsa Id, returns the key. Convenience function to avoid having to type <C::Key as AsId>::from_id
.
impl HasIngredientsFor<compile_effect> for Jar
fn ingredient(&self) -> &<compile_effect as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <compile_effect as IngredientsFor>::Ingredients
impl IngredientsFor for compile_effect
type Ingredients = compile_effect
type Jar = Jar
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
Auto Trait Implementations
impl !Freeze for compile_effect
impl !RefUnwindSafe for compile_effect
impl Send for compile_effect
impl Sync for compile_effect
impl Unpin for compile_effect
impl UnwindSafe for compile_effect
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Module srclang::compiler::text
Structs
Document
:Position
: Represents a position in the source code.SourceMap
: Represents the source map of the program.SourceProgram
: Represents the source program text.Span
: Represents a span of text.SpanOverlap
:Spanned
: Represents a spanned piece of code.__PositionData
: Internal struct used for interned item__SourceMapConfig
:__SpanData
: Internal struct used for interned item__SpannedData
: Internal struct used for interned itemcalculate_line_lengths
:to_spans
:
Functions
calculate_line_lengths
:cmp_range
:to_spans
:
Function srclang::compiler::text::calculate_line_lengths
pub fn calculate_line_lengths(db: &dyn Db, src: SourceProgram) -> Vec<usize>
Function srclang::compiler::text::cmp_range
pub fn cmp_range<T: Ord>(a: &Range<T>, b: &Range<T>) -> SpanOverlap
Function srclang::compiler::text::to_spans
pub fn to_spans(db: &dyn Db, src: SourceProgram) -> SourceMap
Struct srclang::compiler::text::Document
pub struct Document(/* private fields */);
Implementations
impl Document
pub fn new(__db: &<Jar as Jar<'_>>::DynDb, url: String, text: Rope) -> Self
pub fn url<'db>(self, __db: &'db <Jar as Jar<'_>>::DynDb) -> String
pub fn text<'db>(self, __db: &'db <Jar as Jar<'_>>::DynDb) -> Rope
pub fn set_text<'db>( self, __db: &'db mut <Jar as Jar<'_>>::DynDb) -> Setter<'db, Document, Rope>
Trait Implementations
impl AsId for Document
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Document
fn clone(&self) -> Document
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Document
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Document
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Document> for Jar
fn ingredient(&self) -> &<Document as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Document as IngredientsFor>::Ingredients
impl Hash for Document
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Document
type Jar = Jar
type Ingredients = (InputFieldIngredient<Document, String>, InputFieldIngredient<Document, Rope>, InputIngredient<Document>)
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for Document
fn cmp(&self, other: &Document) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Document
fn eq(&self, other: &Document) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Document
fn partial_cmp(&self, other: &Document) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Documentwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for Document
impl Eq for Document
impl StructuralPartialEq for Document
Auto Trait Implementations
impl Freeze for Document
impl RefUnwindSafe for Document
impl Send for Document
impl Sync for Document
impl Unpin for Document
impl UnwindSafe for Document
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::text::Position
pub struct Position(/* private fields */);
Represents a position in the source code.
Implementations
impl Position
pub fn line(self, db: &<Jar as Jar<'_>>::DynDb) -> usize
pub fn column(self, db: &<Jar as Jar<'_>>::DynDb) -> usize
pub fn new(db: &<Jar as Jar<'_>>::DynDb, line: usize, column: usize) -> Self
Trait Implementations
impl AsId for Position
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Position
fn clone(&self) -> Position
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Position
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Position
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Position> for Jar
fn ingredient(&self) -> &<Position as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Position as IngredientsFor>::Ingredients
impl Hash for Position
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Position
type Jar = Jar
type Ingredients = InternedIngredient<Position, __PositionData>
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: JarFromJars<Self::Jar>,
impl Ord for Position
fn cmp(&self, other: &Position) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Position
fn eq(&self, other: &Position) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Position
fn partial_cmp(&self, other: &Position) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Positionwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for Position
impl Eq for Position
impl StructuralPartialEq for Position
Auto Trait Implementations
impl Freeze for Position
impl RefUnwindSafe for Position
impl Send for Position
impl Sync for Position
impl Unpin for Position
impl UnwindSafe for Position
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::text::SourceMap
pub struct SourceMap(/* private fields */);
Represents the source map of the program.
Implementations
impl SourceMap
pub fn new(__db: &<Jar as Jar<'_>>::DynDb, tokens: Vec<Spanned>) -> Self
pub fn tokens<'db>( self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db Vec<Spanned>
Trait Implementations
impl AsId for SourceMap
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for SourceMap
fn clone(&self) -> SourceMap
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for SourceMap
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for SourceMap
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<SourceMap> for Jar
fn ingredient(&self) -> &<SourceMap as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <SourceMap as IngredientsFor>::Ingredients
impl Hash for SourceMap
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for SourceMap
type Jar = Jar
type Ingredients = (TrackedStructIngredient<__SourceMapConfig>, [TrackedFieldIngredient<__SourceMapConfig>; 1])
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for SourceMap
fn cmp(&self, other: &SourceMap) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for SourceMap
fn eq(&self, other: &SourceMap) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for SourceMap
fn partial_cmp(&self, other: &SourceMap) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for SourceMapwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(db: &DB, index: IngredientIndex)
impl<DB> TrackedStructInDb<DB> for SourceMapwhere DB: ?Sized + DbWithJar<Jar>,
fn database_key_index(self, db: &DB) -> DatabaseKeyIndex
Converts the identifier for this tracked struct into a DatabaseKeyIndex
.
impl Copy for SourceMap
impl Eq for SourceMap
impl StructuralPartialEq for SourceMap
Auto Trait Implementations
impl Freeze for SourceMap
impl RefUnwindSafe for SourceMap
impl Send for SourceMap
impl Sync for SourceMap
impl Unpin for SourceMap
impl UnwindSafe for SourceMap
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::text::SourceProgram
pub struct SourceProgram(/* private fields */);
Represents the source program text.
Implementations
impl SourceProgram
pub fn new(__db: &<Jar as Jar<'_>>::DynDb, url: String, text: String) -> Self
pub fn url<'db>(self, __db: &'db <Jar as Jar<'_>>::DynDb) -> String
pub fn text<'db>(self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db String
pub fn set_text<'db>( self, __db: &'db mut <Jar as Jar<'_>>::DynDb) -> Setter<'db, SourceProgram, String>
Trait Implementations
impl AsId for SourceProgram
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for SourceProgram
fn clone(&self) -> SourceProgram
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for SourceProgram
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for SourceProgram
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<SourceProgram> for Jar
fn ingredient(&self) -> &<SourceProgram as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <SourceProgram as IngredientsFor>::Ingredients
impl Hash for SourceProgram
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for SourceProgram
type Jar = Jar
type Ingredients = (InputFieldIngredient<SourceProgram, String>, InputFieldIngredient<SourceProgram, String>, InputIngredient<SourceProgram>)
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for SourceProgram
fn cmp(&self, other: &SourceProgram) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for SourceProgram
fn eq(&self, other: &SourceProgram) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for SourceProgram
fn partial_cmp(&self, other: &SourceProgram) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for SourceProgramwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for SourceProgram
impl Eq for SourceProgram
impl StructuralPartialEq for SourceProgram
Auto Trait Implementations
impl Freeze for SourceProgram
impl RefUnwindSafe for SourceProgram
impl Send for SourceProgram
impl Sync for SourceProgram
impl Unpin for SourceProgram
impl UnwindSafe for SourceProgram
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::text::Span
pub struct Span(/* private fields */);
Represents a span of text.
Implementations
impl Span
pub fn span(self, db: &<Jar as Jar<'_>>::DynDb) -> Range<usize>
pub fn new(db: &<Jar as Jar<'_>>::DynDb, span: Range<usize>) -> Self
Trait Implementations
impl AsId for Span
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Span
fn clone(&self) -> Span
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Span
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Span
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Span> for Jar
fn ingredient(&self) -> &<Span as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Span as IngredientsFor>::Ingredients
impl Hash for Span
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Span
type Jar = Jar
type Ingredients = InternedIngredient<Span, __SpanData>
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: JarFromJars<Self::Jar>,
impl Ord for Span
fn cmp(&self, other: &Span) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Span
fn eq(&self, other: &Span) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Span
fn partial_cmp(&self, other: &Span) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Spanwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for Span
impl Eq for Span
impl StructuralPartialEq for Span
Auto Trait Implementations
impl Freeze for Span
impl RefUnwindSafe for Span
impl Send for Span
impl Sync for Span
impl Unpin for Span
impl UnwindSafe for Span
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::text::SpanOverlap
pub struct SpanOverlap(/* private fields */);
Implementations
impl SpanOverlap
pub const NONE: Self = _
pub const START: Self = _
pub const END: Self = _
pub const BOTH: Self = _
impl SpanOverlap
pub const fn empty() -> Self
Get a flags value with all bits unset.
pub const fn all() -> Self
Get a flags value with all known bits set.
pub const fn bits(&self) -> u8
Get the underlying bits value.
The returned value is exactly the bits set in this flags value.
pub const fn from_bits(bits: u8) -> Option<Self>
Convert from a bits value.
This method will return None
if any unknown bits are set.
pub const fn from_bits_truncate(bits: u8) -> Self
Convert from a bits value, unsetting any unknown bits.
pub const fn from_bits_retain(bits: u8) -> Self
Convert from a bits value exactly.
pub fn from_name(name: &str) -> Option<Self>
Get a flags value with the bits of a flag with the given name set.
This method will return None
if name
is empty or doesn’t correspond to any named flag.
pub const fn is_empty(&self) -> bool
Whether all bits in this flags value are unset.
pub const fn is_all(&self) -> bool
Whether all known bits in this flags value are set.
pub const fn intersects(&self, other: Self) -> bool
Whether any set bits in a source flags value are also set in a target flags value.
pub const fn contains(&self, other: Self) -> bool
Whether all set bits in a source flags value are also set in a target flags value.
pub fn insert(&mut self, other: Self)
The bitwise or ( |
) of the bits in two flags values.
pub fn remove(&mut self, other: Self)
The intersection of a source flags value with the complement of a target flags value ( &!
).
This method is not equivalent to self & !other
when other
has unknown bits set. remove
won’t truncate other
, but the !
operator will.
pub fn toggle(&mut self, other: Self)
The bitwise exclusive-or ( ^
) of the bits in two flags values.
pub fn set(&mut self, other: Self, value: bool)
Call insert
when value
is true
or remove
when value
is false
.
pub const fn intersection(self, other: Self) -> Self
The bitwise and ( &
) of the bits in two flags values.
pub const fn union(self, other: Self) -> Self
The bitwise or ( |
) of the bits in two flags values.
pub const fn difference(self, other: Self) -> Self
The intersection of a source flags value with the complement of a target flags value ( &!
).
This method is not equivalent to self & !other
when other
has unknown bits set. difference
won’t truncate other
, but the !
operator will.
pub const fn symmetric_difference(self, other: Self) -> Self
The bitwise exclusive-or ( ^
) of the bits in two flags values.
pub const fn complement(self) -> Self
The bitwise negation ( !
) of the bits in a flags value, truncating the result.
impl SpanOverlap
pub const fn iter(&self) -> Iter<SpanOverlap>
Yield a set of contained flags values.
Each yielded flags value will correspond to a defined named flag. Any unknown bits will be yielded together as a final flags value.
pub const fn iter_names(&self) -> IterNames<SpanOverlap>
Yield a set of contained named flags values.
This method is like iter
, except only yields bits in contained named flags. Any unknown bits, or bits not corresponding to a contained flag will not be yielded.
Trait Implementations
impl Binary for SpanOverlap
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter.
impl BitAnd for SpanOverlap
fn bitand(self, other: Self) -> Self
The bitwise and ( &
) of the bits in two flags values.
type Output = SpanOverlap
The resulting type after applying the &
operator.
impl BitAndAssign for SpanOverlap
fn bitand_assign(&mut self, other: Self)
The bitwise and ( &
) of the bits in two flags values.
impl BitOr for SpanOverlap
fn bitor(self, other: SpanOverlap) -> Self
The bitwise or ( |
) of the bits in two flags values.
type Output = SpanOverlap
The resulting type after applying the |
operator.
impl BitOrAssign for SpanOverlap
fn bitor_assign(&mut self, other: Self)
The bitwise or ( |
) of the bits in two flags values.
impl BitXor for SpanOverlap
fn bitxor(self, other: Self) -> Self
The bitwise exclusive-or ( ^
) of the bits in two flags values.
type Output = SpanOverlap
The resulting type after applying the ^
operator.
impl BitXorAssign for SpanOverlap
fn bitxor_assign(&mut self, other: Self)
The bitwise exclusive-or ( ^
) of the bits in two flags values.
impl Clone for SpanOverlap
fn clone(&self) -> SpanOverlap
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for SpanOverlap
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Extend<SpanOverlap> for SpanOverlap
fn extend<T: IntoIterator<Item = Self>>(&mut self, iterator: T)
The bitwise or ( |
) of the bits in each flags value.
fn extend_one(&mut self, item: A)
🔬This is a nightly-only experimental API. (extend_one
)Extends a collection with exactly one element.
fn extend_reserve(&mut self, additional: usize)
🔬This is a nightly-only experimental API. (extend_one
)Reserves capacity in a collection for the given number of additional elements. Read more
impl Flags for SpanOverlap
const FLAGS: &'static [Flag<SpanOverlap>] = _
The set of defined flags.
type Bits = u8
The underlying bits type.
fn bits(&self) -> u8
Get the underlying bits value. Read more
fn from_bits_retain(bits: u8) -> SpanOverlap
Convert from a bits value exactly.
fn empty() -> Self
Get a flags value with all bits unset.
fn all() -> Self
Get a flags value with all known bits set.
fn from_bits(bits: Self::Bits) -> Option<Self>
Convert from a bits value. Read more
fn from_bits_truncate(bits: Self::Bits) -> Self
Convert from a bits value, unsetting any unknown bits.
fn from_name(name: &str) -> Option<Self>
Get a flags value with the bits of a flag with the given name set. Read more
fn iter(&self) -> Iter<Self>
Yield a set of contained flags values. Read more
fn iter_names(&self) -> IterNames<Self>
Yield a set of contained named flags values. Read more
fn is_empty(&self) -> bool
Whether all bits in this flags value are unset.
fn is_all(&self) -> bool
Whether all known bits in this flags value are set.
fn intersects(&self, other: Self) -> boolwhere Self: Sized,
Whether any set bits in a source flags value are also set in a target flags value.
fn contains(&self, other: Self) -> boolwhere Self: Sized,
Whether all set bits in a source flags value are also set in a target flags value.
fn insert(&mut self, other: Self)where Self: Sized,
The bitwise or (|
) of the bits in two flags values.
fn remove(&mut self, other: Self)where Self: Sized,
The intersection of a source flags value with the complement of a target flags value (&!
). Read more
fn toggle(&mut self, other: Self)where Self: Sized,
The bitwise exclusive-or (^
) of the bits in two flags values.
fn set(&mut self, other: Self, value: bool)where Self: Sized,
Call Flags::insert
when value
is true
or Flags::remove
when value
is false
.
fn intersection(self, other: Self) -> Self
The bitwise and (&
) of the bits in two flags values.
fn union(self, other: Self) -> Self
The bitwise or (|
) of the bits in two flags values.
fn difference(self, other: Self) -> Self
The intersection of a source flags value with the complement of a target flags value (&!
). Read more
fn symmetric_difference(self, other: Self) -> Self
The bitwise exclusive-or (^
) of the bits in two flags values.
fn complement(self) -> Self
The bitwise negation (!
) of the bits in a flags value, truncating the result.
impl FromIterator<SpanOverlap> for SpanOverlap
fn from_iter<T: IntoIterator<Item = Self>>(iterator: T) -> Self
The bitwise or ( |
) of the bits in each flags value.
impl Hash for SpanOverlap
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IntoIterator for SpanOverlap
type Item = SpanOverlap
The type of the elements being iterated over.
type IntoIter = Iter<SpanOverlap>
Which kind of iterator are we turning this into?
fn into_iter(self) -> Self::IntoIter
Creates an iterator from a value. Read more
impl LowerHex for SpanOverlap
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter.
impl Not for SpanOverlap
fn not(self) -> Self
The bitwise negation ( !
) of the bits in a flags value, truncating the result.
type Output = SpanOverlap
The resulting type after applying the !
operator.
impl Octal for SpanOverlap
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter.
impl Ord for SpanOverlap
fn cmp(&self, other: &SpanOverlap) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for SpanOverlap
fn eq(&self, other: &SpanOverlap) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for SpanOverlap
fn partial_cmp(&self, other: &SpanOverlap) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl PublicFlags for SpanOverlap
type Primitive = u8
The type of the underlying storage.
type Internal = InternalBitFlags
The type of the internal field on the generated flags type.
impl Sub for SpanOverlap
fn sub(self, other: Self) -> Self
The intersection of a source flags value with the complement of a target flags value ( &!
).
This method is not equivalent to self & !other
when other
has unknown bits set. difference
won’t truncate other
, but the !
operator will.
type Output = SpanOverlap
The resulting type after applying the -
operator.
impl SubAssign for SpanOverlap
fn sub_assign(&mut self, other: Self)
The intersection of a source flags value with the complement of a target flags value ( &!
).
This method is not equivalent to self & !other
when other
has unknown bits set. difference
won’t truncate other
, but the !
operator will.
impl UpperHex for SpanOverlap
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter.
impl Copy for SpanOverlap
impl Eq for SpanOverlap
impl StructuralPartialEq for SpanOverlap
Auto Trait Implementations
impl Freeze for SpanOverlap
impl RefUnwindSafe for SpanOverlap
impl Send for SpanOverlap
impl Sync for SpanOverlap
impl Unpin for SpanOverlap
impl UnwindSafe for SpanOverlap
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
Struct srclang::compiler::text::Spanned
pub struct Spanned(/* private fields */);
Represents a spanned piece of code.
Implementations
impl Spanned
pub fn span<'db>(self, db: &'db <Jar as Jar<'_>>::DynDb) -> &'db Span
pub fn src<'db>(self, db: &'db <Jar as Jar<'_>>::DynDb) -> &'db SourceProgram
pub fn pos<'db>(self, db: &'db <Jar as Jar<'_>>::DynDb) -> &'db Position
pub fn new( db: &<Jar as Jar<'_>>::DynDb, span: Span, src: SourceProgram, pos: Position) -> Self
Trait Implementations
impl AsId for Spanned
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for Spanned
fn clone(&self) -> Spanned
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Spanned
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Spanned
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<Spanned> for Jar
fn ingredient(&self) -> &<Spanned as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Spanned as IngredientsFor>::Ingredients
impl Hash for Spanned
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for Spanned
type Jar = Jar
type Ingredients = InternedIngredient<Spanned, __SpannedData>
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: JarFromJars<Self::Jar>,
impl Ord for Spanned
fn cmp(&self, other: &Spanned) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for Spanned
fn eq(&self, other: &Spanned) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Spanned
fn partial_cmp(&self, other: &Spanned) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for Spannedwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for Spanned
impl Eq for Spanned
impl StructuralPartialEq for Spanned
Auto Trait Implementations
impl Freeze for Spanned
impl RefUnwindSafe for Spanned
impl Send for Spanned
impl Sync for Spanned
impl Unpin for Spanned
impl UnwindSafe for Spanned
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::compiler::text::__PositionData
pub struct __PositionData { /* private fields */ }
Internal struct used for interned item
Trait Implementations
impl Clone for __PositionData
fn clone(&self) -> __PositionData
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Hash for __PositionData
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl PartialEq for __PositionData
fn eq(&self, other: &__PositionData) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Eq for __PositionData
impl StructuralPartialEq for __PositionData
Auto Trait Implementations
impl Freeze for __PositionData
impl RefUnwindSafe for __PositionData
impl Send for __PositionData
impl Sync for __PositionData
impl Unpin for __PositionData
impl UnwindSafe for __PositionData
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
Struct srclang::compiler::text::__SourceMapConfig
pub struct __SourceMapConfig { /* private fields */ }
Trait Implementations
impl Configuration for __SourceMapConfig
type Id = SourceMap
The id type used to define instances of this struct. The TrackedStructIngredient
contains the interner that will create the id values.
type Fields = (Vec<Spanned>,)
A (possibly empty) tuple of the fields for this struct.
type Revisions = [Revision; 1]
A array of Revision
values, one per each of the value fields. When a struct is re-recreated in a new revision, the corresponding entries for each field are updated to the new revision if their values have changed (or if the field is marked as #[no_eq]
).
fn id_fields(fields: &Self::Fields) -> impl Hash
fn revision(revisions: &Self::Revisions, field_index: u32) -> Revision
Access the revision of a given value field.field_index
will be between 0 and the number of value fields.
fn new_revisions(current_revision: Revision) -> Self::Revisions
Create a new value revision array where each element is set to current_revision
.
fn update_revisions( current_revision_: Revision, old_value_: &Self::Fields, new_value_: &Self::Fields, revisions_: &mut Self::Revisions)
Update an existing value revision array revisions
, given the tuple of the old values (old_value
) and the tuple of the values (new_value
). If a value has changed, then its element is updated to current_revision
.
Auto Trait Implementations
impl Freeze for __SourceMapConfig
impl RefUnwindSafe for __SourceMapConfig
impl Send for __SourceMapConfig
impl Sync for __SourceMapConfig
impl Unpin for __SourceMapConfig
impl UnwindSafe for __SourceMapConfig
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::compiler::text::__SpanData
pub struct __SpanData { /* private fields */ }
Internal struct used for interned item
Trait Implementations
impl Clone for __SpanData
fn clone(&self) -> __SpanData
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Hash for __SpanData
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl PartialEq for __SpanData
fn eq(&self, other: &__SpanData) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Eq for __SpanData
impl StructuralPartialEq for __SpanData
Auto Trait Implementations
impl Freeze for __SpanData
impl RefUnwindSafe for __SpanData
impl Send for __SpanData
impl Sync for __SpanData
impl Unpin for __SpanData
impl UnwindSafe for __SpanData
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
Struct srclang::compiler::text::__SpannedData
pub struct __SpannedData { /* private fields */ }
Internal struct used for interned item
Trait Implementations
impl Clone for __SpannedData
fn clone(&self) -> __SpannedData
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Hash for __SpannedData
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl PartialEq for __SpannedData
fn eq(&self, other: &__SpannedData) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Eq for __SpannedData
impl StructuralPartialEq for __SpannedData
Auto Trait Implementations
impl Freeze for __SpannedData
impl RefUnwindSafe for __SpannedData
impl Send for __SpannedData
impl Sync for __SpannedData
impl Unpin for __SpannedData
impl UnwindSafe for __SpannedData
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
Struct srclang::compiler::text::calculate_line_lengths
pub struct calculate_line_lengths { /* private fields */ }
Implementations
impl calculate_line_lengths
pub fn get<'__db>(db: &'__db dyn Db, src: SourceProgram) -> &'__db Vec<usize>
pub fn set(db: &mut dyn Db, src: SourceProgram, __value: Vec<usize>)
pub fn accumulated<'__db, __A: Accumulator>( db: &'__db dyn Db, src: SourceProgram) -> Vec<<__A as Accumulator>::Data>where <Jar as Jar<'__db>>::DynDb: HasJar<<__A as Accumulator>::Jar>,
Trait Implementations
impl Configuration for calculate_line_lengths
type Jar = Jar
type SalsaStruct = SourceProgram
The “salsa struct type” that this function is associated with. This can be just salsa::Id
for functions that intern their arguments and are not clearly associated with any one salsa struct.
type Key = SourceProgram
What key is used to index the memo. Typically a salsa struct id, but if this memoized function has multiple arguments it will be a salsa::Id
that results from interning those arguments.
type Value = Vec<usize>
The value computed by the function.
const CYCLE_STRATEGY: CycleRecoveryStrategy = salsa::cycle::CycleRecoveryStrategy::Panic
Determines whether this function can recover from being a participant in a cycle (and, if so, how).
fn should_backdate_value(v1: &Self::Value, v2: &Self::Value) -> bool
Invokes after a new result new_value`` has been computed for which an older memoized value existed
old_value`. Returns true if the new value is equal to the older one and hence should be “backdated” (i.e., marked as having last changed in an older revision, even though it was recomputed). Read more
fn execute(__db: &DynDb<'_, Self>, __id: Self::Key) -> Self::Value
Invoked when we need to compute the value for the given key, either because we’ve never computed it before or because the old one relied on inputs that have changed. Read more
fn recover_from_cycle( _db: &DynDb<'_, Self>, _cycle: &Cycle, _key: Self::Key) -> Self::Value
If the cycle strategy is Recover
, then invoked when key
is a participant in a cycle to find out what value it should have. Read more
fn key_from_id(id: Id) -> Self::Key
Given a salsa Id, returns the key. Convenience function to avoid having to type <C::Key as AsId>::from_id
.
impl HasIngredientsFor<calculate_line_lengths> for Jar
fn ingredient(&self) -> &<calculate_line_lengths as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <calculate_line_lengths as IngredientsFor>::Ingredients
impl IngredientsFor for calculate_line_lengths
type Ingredients = calculate_line_lengths
type Jar = Jar
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
Auto Trait Implementations
impl !Freeze for calculate_line_lengths
impl !RefUnwindSafe for calculate_line_lengths
impl Send for calculate_line_lengths
impl Sync for calculate_line_lengths
impl Unpin for calculate_line_lengths
impl UnwindSafe for calculate_line_lengths
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::compiler::text::to_spans
pub struct to_spans { /* private fields */ }
Implementations
impl to_spans
pub fn get<'__db>(db: &'__db dyn Db, src: SourceProgram) -> &'__db SourceMap
pub fn set(db: &mut dyn Db, src: SourceProgram, __value: SourceMap)
pub fn accumulated<'__db, __A: Accumulator>( db: &'__db dyn Db, src: SourceProgram) -> Vec<<__A as Accumulator>::Data>where <Jar as Jar<'__db>>::DynDb: HasJar<<__A as Accumulator>::Jar>,
Trait Implementations
impl Configuration for to_spans
type Jar = Jar
type SalsaStruct = SourceProgram
The “salsa struct type” that this function is associated with. This can be just salsa::Id
for functions that intern their arguments and are not clearly associated with any one salsa struct.
type Key = SourceProgram
What key is used to index the memo. Typically a salsa struct id, but if this memoized function has multiple arguments it will be a salsa::Id
that results from interning those arguments.
type Value = SourceMap
The value computed by the function.
const CYCLE_STRATEGY: CycleRecoveryStrategy = salsa::cycle::CycleRecoveryStrategy::Panic
Determines whether this function can recover from being a participant in a cycle (and, if so, how).
fn should_backdate_value(v1: &Self::Value, v2: &Self::Value) -> bool
Invokes after a new result new_value`` has been computed for which an older memoized value existed
old_value`. Returns true if the new value is equal to the older one and hence should be “backdated” (i.e., marked as having last changed in an older revision, even though it was recomputed). Read more
fn execute(__db: &DynDb<'_, Self>, __id: Self::Key) -> Self::Value
Invoked when we need to compute the value for the given key, either because we’ve never computed it before or because the old one relied on inputs that have changed. Read more
fn recover_from_cycle( _db: &DynDb<'_, Self>, _cycle: &Cycle, _key: Self::Key) -> Self::Value
If the cycle strategy is Recover
, then invoked when key
is a participant in a cycle to find out what value it should have. Read more
fn key_from_id(id: Id) -> Self::Key
Given a salsa Id, returns the key. Convenience function to avoid having to type <C::Key as AsId>::from_id
.
impl HasIngredientsFor<to_spans> for Jar
fn ingredient(&self) -> &<to_spans as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <to_spans as IngredientsFor>::Ingredients
impl IngredientsFor for to_spans
type Ingredients = to_spans
type Jar = Jar
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
Auto Trait Implementations
impl !Freeze for to_spans
impl !RefUnwindSafe for to_spans
impl Send for to_spans
impl Sync for to_spans
impl Unpin for to_spans
impl UnwindSafe for to_spans
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Module srclang::lexer
lexer
contains the intermediate representation for the src-lang.
Structs
Lexer
:Location
:Position
:Spanned
:TripleIterator
:
Enums
LexicalError
:Token
:Word
:
Enum srclang::lexer::LexicalError
pub enum LexicalError {
UnexpectedCharacter(char),
UnterminatedString,
InvalidNumberFormat,
InvalidVariableFormat,
UnexpectedEndOfInput,
InvalidStateEmission(State),
}
Variants
UnexpectedCharacter(char)
UnterminatedString
InvalidNumberFormat
InvalidVariableFormat
UnexpectedEndOfInput
InvalidStateEmission(State)
Trait Implementations
impl Clone for LexicalError
fn clone(&self) -> LexicalError
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for LexicalError
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for LexicalError
fn eq(&self, other: &LexicalError) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for LexicalError
Auto Trait Implementations
impl Freeze for LexicalError
impl RefUnwindSafe for LexicalError
impl Send for LexicalError
impl Sync for LexicalError
impl Unpin for LexicalError
impl UnwindSafe for LexicalError
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::lexer::Token
pub enum Token<'input> {
Pipe,
Ampersand,
Semicolon,
Equals,
LessThan,
GreaterThan,
Word(Word<'input>),
String(&'input str),
Comment(&'input str),
Integer(i64),
Float(f64),
Eof,
NewLine,
LeftParen,
RightParen,
LeftBrace,
RightBrace,
LeftBracket,
RightBracket,
Comma,
Dot,
Colon,
Underscore,
Minus,
Plus,
Arrow,
FatArrow,
Divide,
Multiply,
Percent,
Dollar,
Exclamation,
Question,
Tilde,
At,
Caret,
Shebang,
}
Variants
Pipe
Ampersand
Semicolon
Equals
LessThan
GreaterThan
Word(Word<'input>)
String(&'input str)
Comment(&'input str)
Integer(i64)
Float(f64)
Eof
NewLine
LeftParen
RightParen
LeftBrace
RightBrace
LeftBracket
RightBracket
Comma
Dot
Colon
Underscore
Minus
Plus
Arrow
FatArrow
Divide
Multiply
Percent
Dollar
Exclamation
Question
Tilde
At
Caret
Shebang
Trait Implementations
impl<'input> Clone for Token<'input>
fn clone(&self) -> Token<'input> ⓘ
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl<'input> Debug for Token<'input>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for Token<'_>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl<'input> Iterator for Token<'input>
type Item = char
The type of the elements being iterated over.
fn next(&mut self) -> Option<Self::Item>
Advances the iterator and returns the next value. Read more
fn next_chunk<const N: usize>( &mut self ) -> Result<[Self::Item; N], IntoIter<Self::Item, N>>where Self: Sized,
🔬This is a nightly-only experimental API. (iter_next_chunk
)Advances the iterator and returns an array containing the next N
values. Read more1.0.0 ·
fn size_hint(&self) -> (usize, Option<usize>)
Returns the bounds on the remaining length of the iterator. Read more1.0.0 ·
fn count(self) -> usizewhere Self: Sized,
Consumes the iterator, counting the number of iterations and returning it. Read more1.0.0 ·
fn last(self) -> Option<Self::Item>where Self: Sized,
Consumes the iterator, returning the last element. Read more
fn advance_by(&mut self, n: usize) -> Result<(), NonZero<usize>>
🔬This is a nightly-only experimental API. (iter_advance_by
)Advances the iterator by n
elements. Read more1.0.0 ·
fn nth(&mut self, n: usize) -> Option<Self::Item>
Returns the n
th element of the iterator. Read more1.28.0 ·
fn step_by(self, step: usize) -> StepBy<Self>where Self: Sized,
Creates an iterator starting at the same point, but stepping by the given amount at each iteration. Read more1.0.0 ·
fn chain<U>(self, other: U) -> Chain<Self, <U as IntoIterator>::IntoIter>where Self: Sized, U: IntoIterator<Item = Self::Item>,
Takes two iterators and creates a new iterator over both in sequence. Read more1.0.0 ·
fn zip<U>(self, other: U) -> Zip<Self, <U as IntoIterator>::IntoIter>where Self: Sized, U: IntoIterator,
‘Zips up’ two iterators into a single iterator of pairs. Read more
fn intersperse_with<G>(self, separator: G) -> IntersperseWith<Self, G>where Self: Sized, G: FnMut() -> Self::Item,
🔬This is a nightly-only experimental API. (iter_intersperse
)Creates a new iterator which places an item generated by separator
between adjacent items of the original iterator. Read more1.0.0 ·
fn map<B, F>(self, f: F) -> Map<Self, F>where Self: Sized, F: FnMut(Self::Item) -> B,
Takes a closure and creates an iterator which calls that closure on each element. Read more1.21.0 ·
fn for_each<F>(self, f: F)where Self: Sized, F: FnMut(Self::Item),
Calls a closure on each element of an iterator. Read more1.0.0 ·
fn filter<P>(self, predicate: P) -> Filter<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator which uses a closure to determine if an element should be yielded. Read more1.0.0 ·
fn filter_map<B, F>(self, f: F) -> FilterMap<Self, F>where Self: Sized, F: FnMut(Self::Item) -> Option<B>,
Creates an iterator that both filters and maps. Read more1.0.0 ·
fn enumerate(self) -> Enumerate<Self>where Self: Sized,
Creates an iterator which gives the current iteration count as well as the next value. Read more1.0.0 ·
fn peekable(self) -> Peekable<Self>where Self: Sized,
Creates an iterator which can use the peek
and peek_mut
methods to look at the next element of the iterator without consuming it. See their documentation for more information. Read more1.0.0 ·
fn skip_while<P>(self, predicate: P) -> SkipWhile<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator that skip
s elements based on a predicate. Read more1.0.0 ·
fn take_while<P>(self, predicate: P) -> TakeWhile<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator that yields elements based on a predicate. Read more1.57.0 ·
fn map_while<B, P>(self, predicate: P) -> MapWhile<Self, P>where Self: Sized, P: FnMut(Self::Item) -> Option<B>,
Creates an iterator that both yields elements based on a predicate and maps. Read more1.0.0 ·
fn skip(self, n: usize) -> Skip<Self>where Self: Sized,
Creates an iterator that skips the first n
elements. Read more1.0.0 ·
fn take(self, n: usize) -> Take<Self>where Self: Sized,
Creates an iterator that yields the first n
elements, or fewer if the underlying iterator ends sooner. Read more1.0.0 ·
fn scan<St, B, F>(self, initial_state: St, f: F) -> Scan<Self, St, F>where Self: Sized, F: FnMut(&mut St, Self::Item) -> Option<B>,
An iterator adapter which, like fold
, holds internal state, but unlike fold
, produces a new iterator. Read more1.0.0 ·
fn flat_map<U, F>(self, f: F) -> FlatMap<Self, U, F>where Self: Sized, U: IntoIterator, F: FnMut(Self::Item) -> U,
Creates an iterator that works like map, but flattens nested structure. Read more
fn map_windows<F, R, const N: usize>(self, f: F) -> MapWindows<Self, F, N>where Self: Sized, F: FnMut(&[Self::Item; N]) -> R,
🔬This is a nightly-only experimental API. (iter_map_windows
)Calls the given function f
for each contiguous window of size N
overself
and returns an iterator over the outputs of f
. Like slice::windows()
, the windows during mapping overlap as well. Read more1.0.0 ·
fn fuse(self) -> Fuse<Self>where Self: Sized,
Creates an iterator which ends after the first None
. Read more1.0.0 ·
fn inspect<F>(self, f: F) -> Inspect<Self, F>where Self: Sized, F: FnMut(&Self::Item),
Does something with each element of an iterator, passing the value on. Read more1.0.0 ·
fn by_ref(&mut self) -> &mut Selfwhere Self: Sized,
Borrows an iterator, rather than consuming it. Read more1.0.0 ·
fn collect<B>(self) -> Bwhere B: FromIterator<Self::Item>, Self: Sized,
Transforms an iterator into a collection. Read more
fn collect_into<E>(self, collection: &mut E) -> &mut Ewhere E: Extend<Self::Item>, Self: Sized,
🔬This is a nightly-only experimental API. (iter_collect_into
)Collects all the items from an iterator into a collection. Read more1.0.0 ·
fn partition<B, F>(self, f: F) -> (B, B)where Self: Sized, B: Default + Extend<Self::Item>, F: FnMut(&Self::Item) -> bool,
Consumes an iterator, creating two collections from it. Read more
fn is_partitioned<P>(self, predicate: P) -> boolwhere Self: Sized, P: FnMut(Self::Item) -> bool,
🔬This is a nightly-only experimental API. (iter_is_partitioned
)Checks if the elements of this iterator are partitioned according to the given predicate, such that all those that return true
precede all those that return false
. Read more1.27.0 ·
fn try_fold<B, F, R>(&mut self, init: B, f: F) -> Rwhere Self: Sized, F: FnMut(B, Self::Item) -> R, R: Try<Output = B>,
An iterator method that applies a function as long as it returns successfully, producing a single, final value. Read more1.27.0 ·
fn try_for_each<F, R>(&mut self, f: F) -> Rwhere Self: Sized, F: FnMut(Self::Item) -> R, R: Try<Output = ()>,
An iterator method that applies a fallible function to each item in the iterator, stopping at the first error and returning that error. Read more1.0.0 ·
fn fold<B, F>(self, init: B, f: F) -> Bwhere Self: Sized, F: FnMut(B, Self::Item) -> B,
Folds every element into an accumulator by applying an operation, returning the final result. Read more1.51.0 ·
fn reduce<F>(self, f: F) -> Option<Self::Item>where Self: Sized, F: FnMut(Self::Item, Self::Item) -> Self::Item,
Reduces the elements to a single one, by repeatedly applying a reducing operation. Read more
fn try_reduce<F, R>( &mut self, f: F ) -> <<R as Try>::Residual as Residual<Option<<R as Try>::Output>>>::TryTypewhere Self: Sized, F: FnMut(Self::Item, Self::Item) -> R, R: Try<Output = Self::Item>, <R as Try>::Residual: Residual<Option<Self::Item>>,
🔬This is a nightly-only experimental API. (iterator_try_reduce
)Reduces the elements to a single one by repeatedly applying a reducing operation. If the closure returns a failure, the failure is propagated back to the caller immediately. Read more1.0.0 ·
fn all<F>(&mut self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> bool,
Tests if every element of the iterator matches a predicate. Read more1.0.0 ·
fn any<F>(&mut self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> bool,
Tests if any element of the iterator matches a predicate. Read more1.0.0 ·
fn find<P>(&mut self, predicate: P) -> Option<Self::Item>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Searches for an element of an iterator that satisfies a predicate. Read more1.30.0 ·
fn find_map<B, F>(&mut self, f: F) -> Option<B>where Self: Sized, F: FnMut(Self::Item) -> Option<B>,
Applies function to the elements of iterator and returns the first non-none result. Read more
fn try_find<F, R>( &mut self, f: F ) -> <<R as Try>::Residual as Residual<Option<Self::Item>>>::TryTypewhere Self: Sized, F: FnMut(&Self::Item) -> R, R: Try<Output = bool>, <R as Try>::Residual: Residual<Option<Self::Item>>,
🔬This is a nightly-only experimental API. (try_find
)Applies function to the elements of iterator and returns the first true result or the first error. Read more1.0.0 ·
fn position<P>(&mut self, predicate: P) -> Option<usize>where Self: Sized, P: FnMut(Self::Item) -> bool,
Searches for an element in an iterator, returning its index. Read more1.6.0 ·
fn max_by_key<B, F>(self, f: F) -> Option<Self::Item>where B: Ord, Self: Sized, F: FnMut(&Self::Item) -> B,
Returns the element that gives the maximum value from the specified function. Read more1.15.0 ·
fn max_by<F>(self, compare: F) -> Option<Self::Item>where Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> Ordering,
Returns the element that gives the maximum value with respect to the specified comparison function. Read more1.6.0 ·
fn min_by_key<B, F>(self, f: F) -> Option<Self::Item>where B: Ord, Self: Sized, F: FnMut(&Self::Item) -> B,
Returns the element that gives the minimum value from the specified function. Read more1.15.0 ·
fn min_by<F>(self, compare: F) -> Option<Self::Item>where Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> Ordering,
Returns the element that gives the minimum value with respect to the specified comparison function. Read more1.0.0 ·
fn unzip<A, B, FromA, FromB>(self) -> (FromA, FromB)where FromA: Default + Extend<A>, FromB: Default + Extend<B>, Self: Sized + Iterator<Item = (A, B)>,
Converts an iterator of pairs into a pair of containers. Read more1.36.0 ·
fn copied<'a, T>(self) -> Copied<Self>where T: 'a + Copy, Self: Sized + Iterator<Item = &'a T>,
Creates an iterator which copies all of its elements. Read more1.0.0 ·
fn cloned<'a, T>(self) -> Cloned<Self>where T: 'a + Clone, Self: Sized + Iterator<Item = &'a T>,
Creates an iterator which clone
s all of its elements. Read more1.0.0 ·
fn cycle(self) -> Cycle<Self>where Self: Sized + Clone,
Repeats an iterator endlessly. Read more
fn array_chunks<const N: usize>(self) -> ArrayChunks<Self, N>where Self: Sized,
🔬This is a nightly-only experimental API. (iter_array_chunks
)Returns an iterator over N
elements of the iterator at a time. Read more1.11.0 ·
fn sum<S>(self) -> Swhere Self: Sized, S: Sum<Self::Item>,
Sums the elements of an iterator. Read more1.11.0 ·
fn product<P>(self) -> Pwhere Self: Sized, P: Product<Self::Item>,
Iterates over the entire iterator, multiplying all the elements Read more
fn cmp_by<I, F>(self, other: I, cmp: F) -> Orderingwhere Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> Ordering,
🔬This is a nightly-only experimental API. (iter_order_by
)Lexicographically compares the elements of this Iterator
with those of another with respect to the specified comparison function. Read more1.5.0 ·
fn partial_cmp<I>(self, other: I) -> Option<Ordering>where I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Lexicographically compares the PartialOrd
elements of this Iterator
with those of another. The comparison works like short-circuit evaluation, returning a result without comparing the remaining elements. As soon as an order can be determined, the evaluation stops and a result is returned. Read more
fn partial_cmp_by<I, F>(self, other: I, partial_cmp: F) -> Option<Ordering>where Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> Option<Ordering>,
🔬This is a nightly-only experimental API. (iter_order_by
)Lexicographically compares the elements of this Iterator
with those of another with respect to the specified comparison function. Read more1.5.0 ·
fn eq<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialEq<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are equal to those of another. Read more
fn eq_by<I, F>(self, other: I, eq: F) -> boolwhere Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> bool,
🔬This is a nightly-only experimental API. (iter_order_by
)Determines if the elements of this Iterator
are equal to those of another with respect to the specified equality function. Read more1.5.0 ·
fn ne<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialEq<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are not equal to those of another. Read more1.5.0 ·
fn lt<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallyless than those of another. Read more1.5.0 ·
fn le<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallyless or equal to those of another. Read more1.5.0 ·
fn gt<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallygreater than those of another. Read more1.5.0 ·
fn ge<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallygreater than or equal to those of another. Read more
fn is_sorted_by<F>(self, compare: F) -> boolwhere Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> bool,
🔬This is a nightly-only experimental API. (is_sorted
)Checks if the elements of this iterator are sorted using the given comparator function. Read more
fn is_sorted_by_key<F, K>(self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> K, K: PartialOrd,
🔬This is a nightly-only experimental API. (is_sorted
)Checks if the elements of this iterator are sorted using the given key extraction function. Read more
impl<'input> PartialEq for Token<'input>
fn eq(&self, other: &Token<'input>) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl<'input> StructuralPartialEq for Token<'input>
Auto Trait Implementations
impl<'input> Freeze for Token<'input>
impl<'input> RefUnwindSafe for Token<'input>
impl<'input> Send for Token<'input>
impl<'input> Sync for Token<'input>
impl<'input> Unpin for Token<'input>
impl<'input> UnwindSafe for Token<'input>
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<I> IntoIterator for Iwhere I: Iterator,
type Item = <I as Iterator>::Item
The type of the elements being iterated over.
type IntoIter = I
Which kind of iterator are we turning this into?const: unstable ·
fn into_iter(self) -> I
Creates an iterator from a value. Read more
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::lexer::Word
pub enum Word<'input> {
Let,
Const,
Fn,
If,
Else,
Return,
Match,
For,
While,
Break,
Continue,
True,
False,
Null,
Action,
Enum,
Impl,
Import,
None,
Struct,
Effect,
When,
Use,
From,
Where,
Self_,
Pub,
Priv,
Ident(&'input str),
FnIdent(&'input str),
Any(&'input str),
}
Variants
Let
Const
Fn
If
Else
Return
Match
For
While
Break
Continue
True
False
Null
Action
Enum
Impl
Import
None
Struct
Effect
When
Use
From
Where
Self_
Pub
Priv
Ident(&'input str)
FnIdent(&'input str)
Any(&'input str)
Trait Implementations
impl<'input> Clone for Word<'input>
fn clone(&self) -> Word<'input>
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl<'input> Debug for Word<'input>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl<'input> PartialEq for Word<'input>
fn eq(&self, other: &Word<'input>) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl<'input> StructuralPartialEq for Word<'input>
Auto Trait Implementations
impl<'input> Freeze for Word<'input>
impl<'input> RefUnwindSafe for Word<'input>
impl<'input> Send for Word<'input>
impl<'input> Sync for Word<'input>
impl<'input> Unpin for Word<'input>
impl<'input> UnwindSafe for Word<'input>
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::lexer::Lexer
pub struct Lexer<'input> { /* private fields */ }
Implementations
impl<'input> Lexer<'input>
pub fn new(input: &'input str, pos: usize) -> Self
impl<'input> Lexer<'input>
pub fn input(&self) -> &'input str
Trait Implementations
impl<'input> Iterator for Lexer<'input>
type Item = Spanned<Token<'input>>
The type of the elements being iterated over.
fn next(&mut self) -> Option<Self::Item>
Advances the iterator and returns the next value. Read more
fn next_chunk<const N: usize>( &mut self ) -> Result<[Self::Item; N], IntoIter<Self::Item, N>>where Self: Sized,
🔬This is a nightly-only experimental API. (iter_next_chunk
)Advances the iterator and returns an array containing the next N
values. Read more1.0.0 ·
fn size_hint(&self) -> (usize, Option<usize>)
Returns the bounds on the remaining length of the iterator. Read more1.0.0 ·
fn count(self) -> usizewhere Self: Sized,
Consumes the iterator, counting the number of iterations and returning it. Read more1.0.0 ·
fn last(self) -> Option<Self::Item>where Self: Sized,
Consumes the iterator, returning the last element. Read more
fn advance_by(&mut self, n: usize) -> Result<(), NonZero<usize>>
🔬This is a nightly-only experimental API. (iter_advance_by
)Advances the iterator by n
elements. Read more1.0.0 ·
fn nth(&mut self, n: usize) -> Option<Self::Item>
Returns the n
th element of the iterator. Read more1.28.0 ·
fn step_by(self, step: usize) -> StepBy<Self>where Self: Sized,
Creates an iterator starting at the same point, but stepping by the given amount at each iteration. Read more1.0.0 ·
fn chain<U>(self, other: U) -> Chain<Self, <U as IntoIterator>::IntoIter>where Self: Sized, U: IntoIterator<Item = Self::Item>,
Takes two iterators and creates a new iterator over both in sequence. Read more1.0.0 ·
fn zip<U>(self, other: U) -> Zip<Self, <U as IntoIterator>::IntoIter>where Self: Sized, U: IntoIterator,
‘Zips up’ two iterators into a single iterator of pairs. Read more
fn intersperse_with<G>(self, separator: G) -> IntersperseWith<Self, G>where Self: Sized, G: FnMut() -> Self::Item,
🔬This is a nightly-only experimental API. (iter_intersperse
)Creates a new iterator which places an item generated by separator
between adjacent items of the original iterator. Read more1.0.0 ·
fn map<B, F>(self, f: F) -> Map<Self, F>where Self: Sized, F: FnMut(Self::Item) -> B,
Takes a closure and creates an iterator which calls that closure on each element. Read more1.21.0 ·
fn for_each<F>(self, f: F)where Self: Sized, F: FnMut(Self::Item),
Calls a closure on each element of an iterator. Read more1.0.0 ·
fn filter<P>(self, predicate: P) -> Filter<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator which uses a closure to determine if an element should be yielded. Read more1.0.0 ·
fn filter_map<B, F>(self, f: F) -> FilterMap<Self, F>where Self: Sized, F: FnMut(Self::Item) -> Option<B>,
Creates an iterator that both filters and maps. Read more1.0.0 ·
fn enumerate(self) -> Enumerate<Self>where Self: Sized,
Creates an iterator which gives the current iteration count as well as the next value. Read more1.0.0 ·
fn peekable(self) -> Peekable<Self>where Self: Sized,
Creates an iterator which can use the peek
and peek_mut
methods to look at the next element of the iterator without consuming it. See their documentation for more information. Read more1.0.0 ·
fn skip_while<P>(self, predicate: P) -> SkipWhile<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator that skip
s elements based on a predicate. Read more1.0.0 ·
fn take_while<P>(self, predicate: P) -> TakeWhile<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator that yields elements based on a predicate. Read more1.57.0 ·
fn map_while<B, P>(self, predicate: P) -> MapWhile<Self, P>where Self: Sized, P: FnMut(Self::Item) -> Option<B>,
Creates an iterator that both yields elements based on a predicate and maps. Read more1.0.0 ·
fn skip(self, n: usize) -> Skip<Self>where Self: Sized,
Creates an iterator that skips the first n
elements. Read more1.0.0 ·
fn take(self, n: usize) -> Take<Self>where Self: Sized,
Creates an iterator that yields the first n
elements, or fewer if the underlying iterator ends sooner. Read more1.0.0 ·
fn scan<St, B, F>(self, initial_state: St, f: F) -> Scan<Self, St, F>where Self: Sized, F: FnMut(&mut St, Self::Item) -> Option<B>,
An iterator adapter which, like fold
, holds internal state, but unlike fold
, produces a new iterator. Read more1.0.0 ·
fn flat_map<U, F>(self, f: F) -> FlatMap<Self, U, F>where Self: Sized, U: IntoIterator, F: FnMut(Self::Item) -> U,
Creates an iterator that works like map, but flattens nested structure. Read more
fn map_windows<F, R, const N: usize>(self, f: F) -> MapWindows<Self, F, N>where Self: Sized, F: FnMut(&[Self::Item; N]) -> R,
🔬This is a nightly-only experimental API. (iter_map_windows
)Calls the given function f
for each contiguous window of size N
overself
and returns an iterator over the outputs of f
. Like slice::windows()
, the windows during mapping overlap as well. Read more1.0.0 ·
fn fuse(self) -> Fuse<Self>where Self: Sized,
Creates an iterator which ends after the first None
. Read more1.0.0 ·
fn inspect<F>(self, f: F) -> Inspect<Self, F>where Self: Sized, F: FnMut(&Self::Item),
Does something with each element of an iterator, passing the value on. Read more1.0.0 ·
fn by_ref(&mut self) -> &mut Selfwhere Self: Sized,
Borrows an iterator, rather than consuming it. Read more1.0.0 ·
fn collect<B>(self) -> Bwhere B: FromIterator<Self::Item>, Self: Sized,
Transforms an iterator into a collection. Read more
fn collect_into<E>(self, collection: &mut E) -> &mut Ewhere E: Extend<Self::Item>, Self: Sized,
🔬This is a nightly-only experimental API. (iter_collect_into
)Collects all the items from an iterator into a collection. Read more1.0.0 ·
fn partition<B, F>(self, f: F) -> (B, B)where Self: Sized, B: Default + Extend<Self::Item>, F: FnMut(&Self::Item) -> bool,
Consumes an iterator, creating two collections from it. Read more
fn is_partitioned<P>(self, predicate: P) -> boolwhere Self: Sized, P: FnMut(Self::Item) -> bool,
🔬This is a nightly-only experimental API. (iter_is_partitioned
)Checks if the elements of this iterator are partitioned according to the given predicate, such that all those that return true
precede all those that return false
. Read more1.27.0 ·
fn try_fold<B, F, R>(&mut self, init: B, f: F) -> Rwhere Self: Sized, F: FnMut(B, Self::Item) -> R, R: Try<Output = B>,
An iterator method that applies a function as long as it returns successfully, producing a single, final value. Read more1.27.0 ·
fn try_for_each<F, R>(&mut self, f: F) -> Rwhere Self: Sized, F: FnMut(Self::Item) -> R, R: Try<Output = ()>,
An iterator method that applies a fallible function to each item in the iterator, stopping at the first error and returning that error. Read more1.0.0 ·
fn fold<B, F>(self, init: B, f: F) -> Bwhere Self: Sized, F: FnMut(B, Self::Item) -> B,
Folds every element into an accumulator by applying an operation, returning the final result. Read more1.51.0 ·
fn reduce<F>(self, f: F) -> Option<Self::Item>where Self: Sized, F: FnMut(Self::Item, Self::Item) -> Self::Item,
Reduces the elements to a single one, by repeatedly applying a reducing operation. Read more
fn try_reduce<F, R>( &mut self, f: F ) -> <<R as Try>::Residual as Residual<Option<<R as Try>::Output>>>::TryTypewhere Self: Sized, F: FnMut(Self::Item, Self::Item) -> R, R: Try<Output = Self::Item>, <R as Try>::Residual: Residual<Option<Self::Item>>,
🔬This is a nightly-only experimental API. (iterator_try_reduce
)Reduces the elements to a single one by repeatedly applying a reducing operation. If the closure returns a failure, the failure is propagated back to the caller immediately. Read more1.0.0 ·
fn all<F>(&mut self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> bool,
Tests if every element of the iterator matches a predicate. Read more1.0.0 ·
fn any<F>(&mut self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> bool,
Tests if any element of the iterator matches a predicate. Read more1.0.0 ·
fn find<P>(&mut self, predicate: P) -> Option<Self::Item>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Searches for an element of an iterator that satisfies a predicate. Read more1.30.0 ·
fn find_map<B, F>(&mut self, f: F) -> Option<B>where Self: Sized, F: FnMut(Self::Item) -> Option<B>,
Applies function to the elements of iterator and returns the first non-none result. Read more
fn try_find<F, R>( &mut self, f: F ) -> <<R as Try>::Residual as Residual<Option<Self::Item>>>::TryTypewhere Self: Sized, F: FnMut(&Self::Item) -> R, R: Try<Output = bool>, <R as Try>::Residual: Residual<Option<Self::Item>>,
🔬This is a nightly-only experimental API. (try_find
)Applies function to the elements of iterator and returns the first true result or the first error. Read more1.0.0 ·
fn position<P>(&mut self, predicate: P) -> Option<usize>where Self: Sized, P: FnMut(Self::Item) -> bool,
Searches for an element in an iterator, returning its index. Read more1.6.0 ·
fn max_by_key<B, F>(self, f: F) -> Option<Self::Item>where B: Ord, Self: Sized, F: FnMut(&Self::Item) -> B,
Returns the element that gives the maximum value from the specified function. Read more1.15.0 ·
fn max_by<F>(self, compare: F) -> Option<Self::Item>where Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> Ordering,
Returns the element that gives the maximum value with respect to the specified comparison function. Read more1.6.0 ·
fn min_by_key<B, F>(self, f: F) -> Option<Self::Item>where B: Ord, Self: Sized, F: FnMut(&Self::Item) -> B,
Returns the element that gives the minimum value from the specified function. Read more1.15.0 ·
fn min_by<F>(self, compare: F) -> Option<Self::Item>where Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> Ordering,
Returns the element that gives the minimum value with respect to the specified comparison function. Read more1.0.0 ·
fn unzip<A, B, FromA, FromB>(self) -> (FromA, FromB)where FromA: Default + Extend<A>, FromB: Default + Extend<B>, Self: Sized + Iterator<Item = (A, B)>,
Converts an iterator of pairs into a pair of containers. Read more1.36.0 ·
fn copied<'a, T>(self) -> Copied<Self>where T: 'a + Copy, Self: Sized + Iterator<Item = &'a T>,
Creates an iterator which copies all of its elements. Read more1.0.0 ·
fn cloned<'a, T>(self) -> Cloned<Self>where T: 'a + Clone, Self: Sized + Iterator<Item = &'a T>,
Creates an iterator which clone
s all of its elements. Read more
fn array_chunks<const N: usize>(self) -> ArrayChunks<Self, N>where Self: Sized,
🔬This is a nightly-only experimental API. (iter_array_chunks
)Returns an iterator over N
elements of the iterator at a time. Read more1.11.0 ·
fn sum<S>(self) -> Swhere Self: Sized, S: Sum<Self::Item>,
Sums the elements of an iterator. Read more1.11.0 ·
fn product<P>(self) -> Pwhere Self: Sized, P: Product<Self::Item>,
Iterates over the entire iterator, multiplying all the elements Read more
fn cmp_by<I, F>(self, other: I, cmp: F) -> Orderingwhere Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> Ordering,
🔬This is a nightly-only experimental API. (iter_order_by
)Lexicographically compares the elements of this Iterator
with those of another with respect to the specified comparison function. Read more1.5.0 ·
fn partial_cmp<I>(self, other: I) -> Option<Ordering>where I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Lexicographically compares the PartialOrd
elements of this Iterator
with those of another. The comparison works like short-circuit evaluation, returning a result without comparing the remaining elements. As soon as an order can be determined, the evaluation stops and a result is returned. Read more
fn partial_cmp_by<I, F>(self, other: I, partial_cmp: F) -> Option<Ordering>where Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> Option<Ordering>,
🔬This is a nightly-only experimental API. (iter_order_by
)Lexicographically compares the elements of this Iterator
with those of another with respect to the specified comparison function. Read more1.5.0 ·
fn eq<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialEq<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are equal to those of another. Read more
fn eq_by<I, F>(self, other: I, eq: F) -> boolwhere Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> bool,
🔬This is a nightly-only experimental API. (iter_order_by
)Determines if the elements of this Iterator
are equal to those of another with respect to the specified equality function. Read more1.5.0 ·
fn ne<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialEq<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are not equal to those of another. Read more1.5.0 ·
fn lt<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallyless than those of another. Read more1.5.0 ·
fn le<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallyless or equal to those of another. Read more1.5.0 ·
fn gt<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallygreater than those of another. Read more1.5.0 ·
fn ge<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallygreater than or equal to those of another. Read more
fn is_sorted_by<F>(self, compare: F) -> boolwhere Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> bool,
🔬This is a nightly-only experimental API. (is_sorted
)Checks if the elements of this iterator are sorted using the given comparator function. Read more
fn is_sorted_by_key<F, K>(self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> K, K: PartialOrd,
🔬This is a nightly-only experimental API. (is_sorted
)Checks if the elements of this iterator are sorted using the given key extraction function. Read more
Auto Trait Implementations
impl<'input> Freeze for Lexer<'input>
impl<'input> RefUnwindSafe for Lexer<'input>
impl<'input> Send for Lexer<'input>
impl<'input> Sync for Lexer<'input>
impl<'input> Unpin for Lexer<'input>
impl<'input> UnwindSafe for Lexer<'input>
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<I> IntoIterator for Iwhere I: Iterator,
type Item = <I as Iterator>::Item
The type of the elements being iterated over.
type IntoIter = I
Which kind of iterator are we turning this into?const: unstable ·
fn into_iter(self) -> I
Creates an iterator from a value. Read more
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::lexer::Location
pub struct Location {
pub offset: usize,
pub line: usize,
pub col: usize,
}
Fields
offset: usize``line: usize``col: usize
Trait Implementations
impl Clone for Location
fn clone(&self) -> Location
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Location
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Default for Location
fn default() -> Location
Returns the “default value” for a type. Read more
impl From<(usize, usize, usize)> for Location
fn from((offset, line, col): (usize, usize, usize)) -> Self
Converts to this type from the input type.
impl Hash for Location
fn hash<H: Hasher>(&self, state: &mut H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl PartialEq for Location
fn eq(&self, other: &Self) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for Location
fn partial_cmp(&self, other: &Self) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl Copy for Location
impl Eq for Location
Auto Trait Implementations
impl Freeze for Location
impl RefUnwindSafe for Location
impl Send for Location
impl Sync for Location
impl Unpin for Location
impl UnwindSafe for Location
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
Struct srclang::lexer::Position
pub struct Position {
pub line: usize,
pub col: usize,
pub size: usize,
}
Fields
line: usize``col: usize``size: usize
Implementations
impl Position
pub fn new(line: usize, col: usize, size: usize) -> Self
Trait Implementations
impl Clone for Position
fn clone(&self) -> Position
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Position
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Default for Position
fn default() -> Position
Returns the “default value” for a type. Read more
impl Display for Position
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Position
fn eq(&self, other: &Position) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Copy for Position
impl StructuralPartialEq for Position
Auto Trait Implementations
impl Freeze for Position
impl RefUnwindSafe for Position
impl Send for Position
impl Sync for Position
impl Unpin for Position
impl UnwindSafe for Position
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::lexer::Spanned
pub struct Spanned<T, P = Position> {
pub node: T,
pub start: usize,
pub end: usize,
pub pos: P,
}
Fields
node: T``start: usize``end: usize``pos: P
Implementations
impl<T, P> Spanned<T, P>
pub fn new(node: T, start: usize, end: usize, pos: P) -> Self
impl Spanned<Token<'_>>
pub fn len(&self) -> usize
pub fn is_empty(&self) -> bool
Trait Implementations
impl<T: Clone, P: Clone> Clone for Spanned<T, P>
fn clone(&self) -> Spanned<T, P>
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl<T: Debug, P: Debug> Debug for Spanned<T, P>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for Spanned<Token<'_>>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl<T: PartialEq, P: PartialEq> PartialEq for Spanned<T, P>
fn eq(&self, other: &Spanned<T, P>) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl<T, P> StructuralPartialEq for Spanned<T, P>
Auto Trait Implementations
impl<T, P> Freeze for Spanned<T, P>where T: Freeze, P: Freeze,
impl<T, P> RefUnwindSafe for Spanned<T, P>where T: RefUnwindSafe, P: RefUnwindSafe,
impl<T, P> Send for Spanned<T, P>where T: Send, P: Send,
impl<T, P> Sync for Spanned<T, P>where T: Sync, P: Sync,
impl<T, P> Unpin for Spanned<T, P>where T: Unpin, P: Unpin,
impl<T, P> UnwindSafe for Spanned<T, P>where T: UnwindSafe, P: UnwindSafe,
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::lexer::TripleIterator
pub struct TripleIterator<'input>(/* private fields */);
Implementations
impl<'input> TripleIterator<'input>
pub fn new(input: &'input str) -> Self
Trait Implementations
impl<'input> Iterator for TripleIterator<'input>
type Item = (Location, Token<'input>, Location)
The type of the elements being iterated over.
fn next(&mut self) -> Option<Self::Item>
Advances the iterator and returns the next value. Read more
fn next_chunk<const N: usize>( &mut self ) -> Result<[Self::Item; N], IntoIter<Self::Item, N>>where Self: Sized,
🔬This is a nightly-only experimental API. (iter_next_chunk
)Advances the iterator and returns an array containing the next N
values. Read more1.0.0 ·
fn size_hint(&self) -> (usize, Option<usize>)
Returns the bounds on the remaining length of the iterator. Read more1.0.0 ·
fn count(self) -> usizewhere Self: Sized,
Consumes the iterator, counting the number of iterations and returning it. Read more1.0.0 ·
fn last(self) -> Option<Self::Item>where Self: Sized,
Consumes the iterator, returning the last element. Read more
fn advance_by(&mut self, n: usize) -> Result<(), NonZero<usize>>
🔬This is a nightly-only experimental API. (iter_advance_by
)Advances the iterator by n
elements. Read more1.0.0 ·
fn nth(&mut self, n: usize) -> Option<Self::Item>
Returns the n
th element of the iterator. Read more1.28.0 ·
fn step_by(self, step: usize) -> StepBy<Self>where Self: Sized,
Creates an iterator starting at the same point, but stepping by the given amount at each iteration. Read more1.0.0 ·
fn chain<U>(self, other: U) -> Chain<Self, <U as IntoIterator>::IntoIter>where Self: Sized, U: IntoIterator<Item = Self::Item>,
Takes two iterators and creates a new iterator over both in sequence. Read more1.0.0 ·
fn zip<U>(self, other: U) -> Zip<Self, <U as IntoIterator>::IntoIter>where Self: Sized, U: IntoIterator,
‘Zips up’ two iterators into a single iterator of pairs. Read more
fn intersperse_with<G>(self, separator: G) -> IntersperseWith<Self, G>where Self: Sized, G: FnMut() -> Self::Item,
🔬This is a nightly-only experimental API. (iter_intersperse
)Creates a new iterator which places an item generated by separator
between adjacent items of the original iterator. Read more1.0.0 ·
fn map<B, F>(self, f: F) -> Map<Self, F>where Self: Sized, F: FnMut(Self::Item) -> B,
Takes a closure and creates an iterator which calls that closure on each element. Read more1.21.0 ·
fn for_each<F>(self, f: F)where Self: Sized, F: FnMut(Self::Item),
Calls a closure on each element of an iterator. Read more1.0.0 ·
fn filter<P>(self, predicate: P) -> Filter<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator which uses a closure to determine if an element should be yielded. Read more1.0.0 ·
fn filter_map<B, F>(self, f: F) -> FilterMap<Self, F>where Self: Sized, F: FnMut(Self::Item) -> Option<B>,
Creates an iterator that both filters and maps. Read more1.0.0 ·
fn enumerate(self) -> Enumerate<Self>where Self: Sized,
Creates an iterator which gives the current iteration count as well as the next value. Read more1.0.0 ·
fn peekable(self) -> Peekable<Self>where Self: Sized,
Creates an iterator which can use the peek
and peek_mut
methods to look at the next element of the iterator without consuming it. See their documentation for more information. Read more1.0.0 ·
fn skip_while<P>(self, predicate: P) -> SkipWhile<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator that skip
s elements based on a predicate. Read more1.0.0 ·
fn take_while<P>(self, predicate: P) -> TakeWhile<Self, P>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Creates an iterator that yields elements based on a predicate. Read more1.57.0 ·
fn map_while<B, P>(self, predicate: P) -> MapWhile<Self, P>where Self: Sized, P: FnMut(Self::Item) -> Option<B>,
Creates an iterator that both yields elements based on a predicate and maps. Read more1.0.0 ·
fn skip(self, n: usize) -> Skip<Self>where Self: Sized,
Creates an iterator that skips the first n
elements. Read more1.0.0 ·
fn take(self, n: usize) -> Take<Self>where Self: Sized,
Creates an iterator that yields the first n
elements, or fewer if the underlying iterator ends sooner. Read more1.0.0 ·
fn scan<St, B, F>(self, initial_state: St, f: F) -> Scan<Self, St, F>where Self: Sized, F: FnMut(&mut St, Self::Item) -> Option<B>,
An iterator adapter which, like fold
, holds internal state, but unlike fold
, produces a new iterator. Read more1.0.0 ·
fn flat_map<U, F>(self, f: F) -> FlatMap<Self, U, F>where Self: Sized, U: IntoIterator, F: FnMut(Self::Item) -> U,
Creates an iterator that works like map, but flattens nested structure. Read more
fn map_windows<F, R, const N: usize>(self, f: F) -> MapWindows<Self, F, N>where Self: Sized, F: FnMut(&[Self::Item; N]) -> R,
🔬This is a nightly-only experimental API. (iter_map_windows
)Calls the given function f
for each contiguous window of size N
overself
and returns an iterator over the outputs of f
. Like slice::windows()
, the windows during mapping overlap as well. Read more1.0.0 ·
fn fuse(self) -> Fuse<Self>where Self: Sized,
Creates an iterator which ends after the first None
. Read more1.0.0 ·
fn inspect<F>(self, f: F) -> Inspect<Self, F>where Self: Sized, F: FnMut(&Self::Item),
Does something with each element of an iterator, passing the value on. Read more1.0.0 ·
fn by_ref(&mut self) -> &mut Selfwhere Self: Sized,
Borrows an iterator, rather than consuming it. Read more1.0.0 ·
fn collect<B>(self) -> Bwhere B: FromIterator<Self::Item>, Self: Sized,
Transforms an iterator into a collection. Read more
fn collect_into<E>(self, collection: &mut E) -> &mut Ewhere E: Extend<Self::Item>, Self: Sized,
🔬This is a nightly-only experimental API. (iter_collect_into
)Collects all the items from an iterator into a collection. Read more1.0.0 ·
fn partition<B, F>(self, f: F) -> (B, B)where Self: Sized, B: Default + Extend<Self::Item>, F: FnMut(&Self::Item) -> bool,
Consumes an iterator, creating two collections from it. Read more
fn is_partitioned<P>(self, predicate: P) -> boolwhere Self: Sized, P: FnMut(Self::Item) -> bool,
🔬This is a nightly-only experimental API. (iter_is_partitioned
)Checks if the elements of this iterator are partitioned according to the given predicate, such that all those that return true
precede all those that return false
. Read more1.27.0 ·
fn try_fold<B, F, R>(&mut self, init: B, f: F) -> Rwhere Self: Sized, F: FnMut(B, Self::Item) -> R, R: Try<Output = B>,
An iterator method that applies a function as long as it returns successfully, producing a single, final value. Read more1.27.0 ·
fn try_for_each<F, R>(&mut self, f: F) -> Rwhere Self: Sized, F: FnMut(Self::Item) -> R, R: Try<Output = ()>,
An iterator method that applies a fallible function to each item in the iterator, stopping at the first error and returning that error. Read more1.0.0 ·
fn fold<B, F>(self, init: B, f: F) -> Bwhere Self: Sized, F: FnMut(B, Self::Item) -> B,
Folds every element into an accumulator by applying an operation, returning the final result. Read more1.51.0 ·
fn reduce<F>(self, f: F) -> Option<Self::Item>where Self: Sized, F: FnMut(Self::Item, Self::Item) -> Self::Item,
Reduces the elements to a single one, by repeatedly applying a reducing operation. Read more
fn try_reduce<F, R>( &mut self, f: F ) -> <<R as Try>::Residual as Residual<Option<<R as Try>::Output>>>::TryTypewhere Self: Sized, F: FnMut(Self::Item, Self::Item) -> R, R: Try<Output = Self::Item>, <R as Try>::Residual: Residual<Option<Self::Item>>,
🔬This is a nightly-only experimental API. (iterator_try_reduce
)Reduces the elements to a single one by repeatedly applying a reducing operation. If the closure returns a failure, the failure is propagated back to the caller immediately. Read more1.0.0 ·
fn all<F>(&mut self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> bool,
Tests if every element of the iterator matches a predicate. Read more1.0.0 ·
fn any<F>(&mut self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> bool,
Tests if any element of the iterator matches a predicate. Read more1.0.0 ·
fn find<P>(&mut self, predicate: P) -> Option<Self::Item>where Self: Sized, P: FnMut(&Self::Item) -> bool,
Searches for an element of an iterator that satisfies a predicate. Read more1.30.0 ·
fn find_map<B, F>(&mut self, f: F) -> Option<B>where Self: Sized, F: FnMut(Self::Item) -> Option<B>,
Applies function to the elements of iterator and returns the first non-none result. Read more
fn try_find<F, R>( &mut self, f: F ) -> <<R as Try>::Residual as Residual<Option<Self::Item>>>::TryTypewhere Self: Sized, F: FnMut(&Self::Item) -> R, R: Try<Output = bool>, <R as Try>::Residual: Residual<Option<Self::Item>>,
🔬This is a nightly-only experimental API. (try_find
)Applies function to the elements of iterator and returns the first true result or the first error. Read more1.0.0 ·
fn position<P>(&mut self, predicate: P) -> Option<usize>where Self: Sized, P: FnMut(Self::Item) -> bool,
Searches for an element in an iterator, returning its index. Read more1.6.0 ·
fn max_by_key<B, F>(self, f: F) -> Option<Self::Item>where B: Ord, Self: Sized, F: FnMut(&Self::Item) -> B,
Returns the element that gives the maximum value from the specified function. Read more1.15.0 ·
fn max_by<F>(self, compare: F) -> Option<Self::Item>where Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> Ordering,
Returns the element that gives the maximum value with respect to the specified comparison function. Read more1.6.0 ·
fn min_by_key<B, F>(self, f: F) -> Option<Self::Item>where B: Ord, Self: Sized, F: FnMut(&Self::Item) -> B,
Returns the element that gives the minimum value from the specified function. Read more1.15.0 ·
fn min_by<F>(self, compare: F) -> Option<Self::Item>where Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> Ordering,
Returns the element that gives the minimum value with respect to the specified comparison function. Read more1.0.0 ·
fn unzip<A, B, FromA, FromB>(self) -> (FromA, FromB)where FromA: Default + Extend<A>, FromB: Default + Extend<B>, Self: Sized + Iterator<Item = (A, B)>,
Converts an iterator of pairs into a pair of containers. Read more1.36.0 ·
fn copied<'a, T>(self) -> Copied<Self>where T: 'a + Copy, Self: Sized + Iterator<Item = &'a T>,
Creates an iterator which copies all of its elements. Read more1.0.0 ·
fn cloned<'a, T>(self) -> Cloned<Self>where T: 'a + Clone, Self: Sized + Iterator<Item = &'a T>,
Creates an iterator which clone
s all of its elements. Read more
fn array_chunks<const N: usize>(self) -> ArrayChunks<Self, N>where Self: Sized,
🔬This is a nightly-only experimental API. (iter_array_chunks
)Returns an iterator over N
elements of the iterator at a time. Read more1.11.0 ·
fn sum<S>(self) -> Swhere Self: Sized, S: Sum<Self::Item>,
Sums the elements of an iterator. Read more1.11.0 ·
fn product<P>(self) -> Pwhere Self: Sized, P: Product<Self::Item>,
Iterates over the entire iterator, multiplying all the elements Read more
fn cmp_by<I, F>(self, other: I, cmp: F) -> Orderingwhere Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> Ordering,
🔬This is a nightly-only experimental API. (iter_order_by
)Lexicographically compares the elements of this Iterator
with those of another with respect to the specified comparison function. Read more1.5.0 ·
fn partial_cmp<I>(self, other: I) -> Option<Ordering>where I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Lexicographically compares the PartialOrd
elements of this Iterator
with those of another. The comparison works like short-circuit evaluation, returning a result without comparing the remaining elements. As soon as an order can be determined, the evaluation stops and a result is returned. Read more
fn partial_cmp_by<I, F>(self, other: I, partial_cmp: F) -> Option<Ordering>where Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> Option<Ordering>,
🔬This is a nightly-only experimental API. (iter_order_by
)Lexicographically compares the elements of this Iterator
with those of another with respect to the specified comparison function. Read more1.5.0 ·
fn eq<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialEq<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are equal to those of another. Read more
fn eq_by<I, F>(self, other: I, eq: F) -> boolwhere Self: Sized, I: IntoIterator, F: FnMut(Self::Item, <I as IntoIterator>::Item) -> bool,
🔬This is a nightly-only experimental API. (iter_order_by
)Determines if the elements of this Iterator
are equal to those of another with respect to the specified equality function. Read more1.5.0 ·
fn ne<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialEq<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are not equal to those of another. Read more1.5.0 ·
fn lt<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallyless than those of another. Read more1.5.0 ·
fn le<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallyless or equal to those of another. Read more1.5.0 ·
fn gt<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallygreater than those of another. Read more1.5.0 ·
fn ge<I>(self, other: I) -> boolwhere I: IntoIterator, Self::Item: PartialOrd<<I as IntoIterator>::Item>, Self: Sized,
Determines if the elements of this Iterator
are lexicographicallygreater than or equal to those of another. Read more
fn is_sorted_by<F>(self, compare: F) -> boolwhere Self: Sized, F: FnMut(&Self::Item, &Self::Item) -> bool,
🔬This is a nightly-only experimental API. (is_sorted
)Checks if the elements of this iterator are sorted using the given comparator function. Read more
fn is_sorted_by_key<F, K>(self, f: F) -> boolwhere Self: Sized, F: FnMut(Self::Item) -> K, K: PartialOrd,
🔬This is a nightly-only experimental API. (is_sorted
)Checks if the elements of this iterator are sorted using the given key extraction function. Read more
Auto Trait Implementations
impl<'input> Freeze for TripleIterator<'input>
impl<'input> RefUnwindSafe for TripleIterator<'input>
impl<'input> Send for TripleIterator<'input>
impl<'input> Sync for TripleIterator<'input>
impl<'input> Unpin for TripleIterator<'input>
impl<'input> UnwindSafe for TripleIterator<'input>
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<I> IntoIterator for Iwhere I: Iterator,
type Item = <I as Iterator>::Item
The type of the elements being iterated over.
type IntoIter = I
Which kind of iterator are we turning this into?const: unstable ·
fn into_iter(self) -> I
Creates an iterator from a value. Read more
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Macro srclang::span
macro_rules! span {
($coord:expr, $expr:expr) => { ... };
($start:expr, $expr:expr, $end:expr) => { ... };
}
A macro to create a Spanned<T>
object Heavily used in `src.lalrpop to create a Spanned object
Module srclang::ops
ops
contains the operations tree traversal operations for the src-lang.
Modules
traversal
: This module contains the srclang ops.
Module srclang::ops::traversal
This module contains the srclang ops.
Enums
Control
: The result of a traversal operation.
Enum srclang::ops::traversal::Control
pub enum Control {
Continue,
Break,
}
The result of a traversal operation.
Variants
Continue
Continue the traversal.
Break
Stop the traversal.
Trait Implementations
impl Debug for Control
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Control
fn eq(&self, other: &Control) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Eq for Control
impl StructuralPartialEq for Control
Auto Trait Implementations
impl Freeze for Control
impl RefUnwindSafe for Control
impl Send for Control
impl Sync for Control
impl Unpin for Control
impl UnwindSafe for Control
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Module srclang::parser
parser
contains the parser for the src-lang, which is written in LALRPOP.
Modules
ast
:span
:src
:
Module srclang::parser::ast
Structs
Array
:BinaryOperation
:Binding
:Block
:BranchDef
:EffectDef
:FieldAccess
:FieldDef
:FnCall
:FnDef
:FnIdent
:Ident
:ImplDef
:KeywordAndVisibility
:Module
:Prototype
:StringLit
:StructDef
:Tuple
:UseDef
:
Enums
FnArg
:Keyword
:Literal
:Node
:Operator
:Value
:Visibility
:Whitespace
:
Constants
ANON_FN_NAME
:
Constant srclang::parser::ast::ANON_FN_NAME
pub const ANON_FN_NAME: &str = "anonymous";
Enum srclang::parser::ast::FnArg
pub enum FnArg {
Reciever,
Field(Spanned<FieldDef>),
}
Variants
Reciever
Field(Spanned<FieldDef>)
Trait Implementations
impl Clone for FnArg
fn clone(&self) -> FnArg
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for FnArg
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for FnArg
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for FnArg
fn eq(&self, other: &FnArg) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for FnArg
Auto Trait Implementations
impl Freeze for FnArg
impl RefUnwindSafe for FnArg
impl Send for FnArg
impl Sync for FnArg
impl Unpin for FnArg
impl UnwindSafe for FnArg
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::parser::ast::Keyword
pub enum Keyword {
None,
Some,
Let,
Public,
Private,
Fn,
If,
Else,
Match,
Arrow,
Struct,
SelfValue,
When,
Effect,
Impl,
Use,
From,
Where,
Self_,
}
Variants
None
Some
Let
Public
Private
Fn
If
Else
Match
Arrow
Struct
SelfValue
When
Effect
Impl
Use
From
Where
Self_
Trait Implementations
impl Clone for Keyword
fn clone(&self) -> Keyword
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Keyword
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for Keyword
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Keyword
fn eq(&self, other: &Keyword) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Keyword
Auto Trait Implementations
impl Freeze for Keyword
impl RefUnwindSafe for Keyword
impl Send for Keyword
impl Sync for Keyword
impl Unpin for Keyword
impl UnwindSafe for Keyword
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::parser::ast::Literal
pub enum Literal {
Bool(bool),
Float(f64),
Integer(i64),
String(String),
}
Variants
Bool(bool)
Float(f64)
Integer(i64)
String(String)
Trait Implementations
impl Clone for Literal
fn clone(&self) -> Literal
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Literal
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Literal
fn eq(&self, other: &Literal) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Literal
Auto Trait Implementations
impl Freeze for Literal
impl RefUnwindSafe for Literal
impl Send for Literal
impl Sync for Literal
impl Unpin for Literal
impl UnwindSafe for Literal
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::parser::ast::Node
pub enum Node {
BinaryExpression(BinaryOperation),
Bool(bool),
Integer(i64),
Float(f64),
Ident(Spanned<Ident>),
Binding(Binding),
FnCall(FnCall),
String(String),
FnDef(FnDef),
EffectDef(EffectDef),
StructDef(StructDef),
UseDef(UseDef),
Keyword(Keyword),
ImplDef(ImplDef),
Branch(BranchDef),
FieldAccess(FieldAccess),
Visibility(Visibility),
Error,
}
Variants
BinaryExpression(BinaryOperation)
Bool(bool)
Integer(i64)
Float(f64)
Ident(Spanned<Ident>)
Binding(Binding)
FnCall(FnCall)
String(String)
FnDef(FnDef)
EffectDef(EffectDef)
StructDef(StructDef)
UseDef(UseDef)
Keyword(Keyword)
ImplDef(ImplDef)
Branch(BranchDef)
FieldAccess(FieldAccess)
Visibility(Visibility)
Error
Trait Implementations
impl Clone for Node
fn clone(&self) -> Node
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Node
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for Node
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Node
fn eq(&self, other: &Node) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl Eq for Node
impl StructuralPartialEq for Node
Auto Trait Implementations
impl Freeze for Node
impl RefUnwindSafe for Node
impl Send for Node
impl Sync for Node
impl Unpin for Node
impl UnwindSafe for Node
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::parser::ast::Operator
pub enum Operator {
Add,
Sub,
Mul,
Div,
Modulo,
Increment,
Decrement,
Maybe,
Not,
Neg,
Dot,
Arrow,
FatArrow,
DoubleColon,
}
Variants
Add
Sub
Mul
Div
Modulo
Increment
Decrement
Maybe
Not
Neg
Dot
Arrow
FatArrow
DoubleColon
Trait Implementations
impl Clone for Operator
fn clone(&self) -> Operator
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Operator
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for Operator
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Operator
fn eq(&self, other: &Operator) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Operator
Auto Trait Implementations
impl Freeze for Operator
impl RefUnwindSafe for Operator
impl Send for Operator
impl Sync for Operator
impl Unpin for Operator
impl UnwindSafe for Operator
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::parser::ast::Value
pub enum Value {
Literal(Literal),
Ident(Ident),
}
Variants
Literal(Literal)
Ident(Ident)
Trait Implementations
impl Clone for Value
fn clone(&self) -> Value
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Value
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Value
fn eq(&self, other: &Value) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Value
Auto Trait Implementations
impl Freeze for Value
impl RefUnwindSafe for Value
impl Send for Value
impl Sync for Value
impl Unpin for Value
impl UnwindSafe for Value
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::parser::ast::Visibility
pub enum Visibility {
Private,
Public,
}
Variants
Private
Public
Trait Implementations
impl Clone for Visibility
fn clone(&self) -> Visibility
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Visibility
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Default for Visibility
fn default() -> Visibility
Returns the “default value” for a type. Read more
impl Display for Visibility
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Visibility
fn eq(&self, other: &Visibility) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Visibility
Auto Trait Implementations
impl Freeze for Visibility
impl RefUnwindSafe for Visibility
impl Send for Visibility
impl Sync for Visibility
impl Unpin for Visibility
impl UnwindSafe for Visibility
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Enum srclang::parser::ast::Whitespace
pub enum Whitespace {
Space,
Tab,
Newline,
}
Variants
Space
Tab
Newline
Trait Implementations
impl Clone for Whitespace
fn clone(&self) -> Whitespace
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Whitespace
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Whitespace
fn eq(&self, other: &Whitespace) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Whitespace
Auto Trait Implementations
impl Freeze for Whitespace
impl RefUnwindSafe for Whitespace
impl Send for Whitespace
impl Sync for Whitespace
impl Unpin for Whitespace
impl UnwindSafe for Whitespace
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::Array
pub struct Array<T>(pub Vec<T>);
Tuple Fields
0: Vec<T>
Trait Implementations
impl<T: Clone> Clone for Array<T>
fn clone(&self) -> Array<T>
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl<T: Debug> Debug for Array<T>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl<T: PartialEq> PartialEq for Array<T>
fn eq(&self, other: &Array<T>) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl<T> StructuralPartialEq for Array<T>
Auto Trait Implementations
impl<T> Freeze for Array<T>
impl<T> RefUnwindSafe for Array<T>where T: RefUnwindSafe,
impl<T> Send for Array<T>where T: Send,
impl<T> Sync for Array<T>where T: Sync,
impl<T> Unpin for Array<T>where T: Unpin,
impl<T> UnwindSafe for Array<T>where T: UnwindSafe,
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::BinaryOperation
pub struct BinaryOperation {
pub lhs: Box<Spanned<Node>>,
pub op: Operator,
pub rhs: Box<Spanned<Node>>,
}
Fields
lhs: Box<Spanned<Node>>``op: Operator``rhs: Box<Spanned<Node>>
Trait Implementations
impl Clone for BinaryOperation
fn clone(&self) -> BinaryOperation
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for BinaryOperation
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for BinaryOperation
fn eq(&self, other: &BinaryOperation) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for BinaryOperation
Auto Trait Implementations
impl Freeze for BinaryOperation
impl RefUnwindSafe for BinaryOperation
impl Send for BinaryOperation
impl Sync for BinaryOperation
impl Unpin for BinaryOperation
impl UnwindSafe for BinaryOperation
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::Binding
pub struct Binding(pub Spanned<Ident>, pub Box<Spanned<Node>>);
Tuple Fields
0: Spanned<Ident>``1: Box<Spanned<Node>>
Trait Implementations
impl Clone for Binding
fn clone(&self) -> Binding
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Binding
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Binding
fn eq(&self, other: &Binding) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Binding
Auto Trait Implementations
impl Freeze for Binding
impl RefUnwindSafe for Binding
impl Send for Binding
impl Sync for Binding
impl Unpin for Binding
impl UnwindSafe for Binding
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::Block
pub struct Block<T>(pub Vec<T>);
Tuple Fields
0: Vec<T>
Trait Implementations
impl<T: Clone> Clone for Block<T>
fn clone(&self) -> Block<T>
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl<T: Debug> Debug for Block<T>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl<T: PartialEq> PartialEq for Block<T>
fn eq(&self, other: &Block<T>) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl<T> StructuralPartialEq for Block<T>
Auto Trait Implementations
impl<T> Freeze for Block<T>
impl<T> RefUnwindSafe for Block<T>where T: RefUnwindSafe,
impl<T> Send for Block<T>where T: Send,
impl<T> Sync for Block<T>where T: Sync,
impl<T> Unpin for Block<T>where T: Unpin,
impl<T> UnwindSafe for Block<T>where T: UnwindSafe,
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::BranchDef
pub struct BranchDef(pub Box<Spanned<Node>>, pub Vec<(Spanned<Node>, Block<Spanned<Node>>)>);
Tuple Fields
0: Box<Spanned<Node>>``1: Vec<(Spanned<Node>, Block<Spanned<Node>>)>
Trait Implementations
impl Clone for BranchDef
fn clone(&self) -> BranchDef
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for BranchDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for BranchDef
fn eq(&self, other: &BranchDef) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for BranchDef
Auto Trait Implementations
impl Freeze for BranchDef
impl RefUnwindSafe for BranchDef
impl Send for BranchDef
impl Sync for BranchDef
impl Unpin for BranchDef
impl UnwindSafe for BranchDef
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::EffectDef
pub struct EffectDef(pub Spanned<KeywordAndVisibility>, pub Spanned<Ident>, pub Vec<Spanned<Ident>>, pub Block<Spanned<Prototype>>);
Tuple Fields
0: Spanned<KeywordAndVisibility>``1: Spanned<Ident>``2: Vec<Spanned<Ident>>``3: Block<Spanned<Prototype>>
Trait Implementations
impl Clone for EffectDef
fn clone(&self) -> EffectDef
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for EffectDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for EffectDef
fn eq(&self, other: &EffectDef) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for EffectDef
Auto Trait Implementations
impl Freeze for EffectDef
impl RefUnwindSafe for EffectDef
impl Send for EffectDef
impl Sync for EffectDef
impl Unpin for EffectDef
impl UnwindSafe for EffectDef
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::FieldAccess
pub struct FieldAccess(pub Box<Spanned<Node>>, pub Box<Spanned<Node>>);
Tuple Fields
0: Box<Spanned<Node>>``1: Box<Spanned<Node>>
Trait Implementations
impl Clone for FieldAccess
fn clone(&self) -> FieldAccess
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for FieldAccess
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for FieldAccess
fn eq(&self, other: &FieldAccess) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for FieldAccess
Auto Trait Implementations
impl Freeze for FieldAccess
impl RefUnwindSafe for FieldAccess
impl Send for FieldAccess
impl Sync for FieldAccess
impl Unpin for FieldAccess
impl UnwindSafe for FieldAccess
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::FieldDef
pub struct FieldDef(pub Spanned<Visibility>, pub Spanned<Ident>, pub Spanned<Ident>);
Tuple Fields
0: Spanned<Visibility>``1: Spanned<Ident>``2: Spanned<Ident>
Trait Implementations
impl Clone for FieldDef
fn clone(&self) -> FieldDef
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for FieldDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for FieldDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for FieldDef
fn eq(&self, other: &FieldDef) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for FieldDef
Auto Trait Implementations
impl Freeze for FieldDef
impl RefUnwindSafe for FieldDef
impl Send for FieldDef
impl Sync for FieldDef
impl Unpin for FieldDef
impl UnwindSafe for FieldDef
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::FnCall
pub struct FnCall(pub Spanned<Ident>, pub Vec<Spanned<Node>>);
Tuple Fields
0: Spanned<Ident>``1: Vec<Spanned<Node>>
Trait Implementations
impl Clone for FnCall
fn clone(&self) -> FnCall
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for FnCall
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for FnCall
fn eq(&self, other: &FnCall) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for FnCall
Auto Trait Implementations
impl Freeze for FnCall
impl RefUnwindSafe for FnCall
impl Send for FnCall
impl Sync for FnCall
impl Unpin for FnCall
impl UnwindSafe for FnCall
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::FnDef
pub struct FnDef(pub Spanned<KeywordAndVisibility>, pub Spanned<Prototype>, pub Block<Spanned<Node>>);
Tuple Fields
0: Spanned<KeywordAndVisibility>``1: Spanned<Prototype>``2: Block<Spanned<Node>>
Trait Implementations
impl Clone for FnDef
fn clone(&self) -> FnDef
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for FnDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for FnDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for FnDef
fn eq(&self, other: &FnDef) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for FnDef
Auto Trait Implementations
impl Freeze for FnDef
impl RefUnwindSafe for FnDef
impl Send for FnDef
impl Sync for FnDef
impl Unpin for FnDef
impl UnwindSafe for FnDef
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::FnIdent
pub struct FnIdent(pub Ident);
Tuple Fields
0: Ident
Trait Implementations
impl Clone for FnIdent
fn clone(&self) -> FnIdent
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for FnIdent
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for FnIdent
fn eq(&self, other: &FnIdent) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for FnIdent
Auto Trait Implementations
impl Freeze for FnIdent
impl RefUnwindSafe for FnIdent
impl Send for FnIdent
impl Sync for FnIdent
impl Unpin for FnIdent
impl UnwindSafe for FnIdent
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::Ident
pub struct Ident(pub String, pub Option<Vec<Spanned<Ident>>>);
Tuple Fields
0: String``1: Option<Vec<Spanned<Ident>>>
Trait Implementations
impl Clone for Ident
fn clone(&self) -> Ident
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Ident
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for Ident
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Ident
fn eq(&self, other: &Ident) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Ident
Auto Trait Implementations
impl Freeze for Ident
impl RefUnwindSafe for Ident
impl Send for Ident
impl Sync for Ident
impl Unpin for Ident
impl UnwindSafe for Ident
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::ImplDef
pub struct ImplDef(pub Spanned<KeywordAndVisibility>, pub Spanned<Ident>, pub Option<Spanned<Ident>>, pub Block<Spanned<Node>>);
Tuple Fields
0: Spanned<KeywordAndVisibility>``1: Spanned<Ident>``2: Option<Spanned<Ident>>``3: Block<Spanned<Node>>
Trait Implementations
impl Clone for ImplDef
fn clone(&self) -> ImplDef
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for ImplDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for ImplDef
fn eq(&self, other: &ImplDef) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for ImplDef
Auto Trait Implementations
impl Freeze for ImplDef
impl RefUnwindSafe for ImplDef
impl Send for ImplDef
impl Sync for ImplDef
impl Unpin for ImplDef
impl UnwindSafe for ImplDef
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::KeywordAndVisibility
pub struct KeywordAndVisibility(pub Spanned<Keyword>, pub Spanned<Visibility>);
Tuple Fields
0: Spanned<Keyword>``1: Spanned<Visibility>
Trait Implementations
impl Clone for KeywordAndVisibility
fn clone(&self) -> KeywordAndVisibility
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for KeywordAndVisibility
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for KeywordAndVisibility
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for KeywordAndVisibility
fn eq(&self, other: &KeywordAndVisibility) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for KeywordAndVisibility
Auto Trait Implementations
impl Freeze for KeywordAndVisibility
impl RefUnwindSafe for KeywordAndVisibility
impl Send for KeywordAndVisibility
impl Sync for KeywordAndVisibility
impl Unpin for KeywordAndVisibility
impl UnwindSafe for KeywordAndVisibility
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::Module
pub struct Module(pub Vec<Spanned<Node>>);
Tuple Fields
0: Vec<Spanned<Node>>
Trait Implementations
impl Clone for Module
fn clone(&self) -> Module
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Module
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Module
fn eq(&self, other: &Module) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Module
Auto Trait Implementations
impl Freeze for Module
impl RefUnwindSafe for Module
impl Send for Module
impl Sync for Module
impl Unpin for Module
impl UnwindSafe for Module
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::Prototype
pub struct Prototype {
pub name: Spanned<Ident>,
pub args: Vec<Spanned<FnArg>>,
pub ret: Option<Spanned<Ident>>,
pub effects: Vec<Spanned<Ident>>,
}
Fields
name: Spanned<Ident>``args: Vec<Spanned<FnArg>>``ret: Option<Spanned<Ident>>``effects: Vec<Spanned<Ident>>
Trait Implementations
impl Clone for Prototype
fn clone(&self) -> Prototype
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for Prototype
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for Prototype
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for Prototype
fn eq(&self, other: &Prototype) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for Prototype
Auto Trait Implementations
impl Freeze for Prototype
impl RefUnwindSafe for Prototype
impl Send for Prototype
impl Sync for Prototype
impl Unpin for Prototype
impl UnwindSafe for Prototype
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::StringLit
pub struct StringLit(pub String);
Tuple Fields
0: String
Trait Implementations
impl Clone for StringLit
fn clone(&self) -> StringLit
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for StringLit
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for StringLit
fn eq(&self, other: &StringLit) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for StringLit
Auto Trait Implementations
impl Freeze for StringLit
impl RefUnwindSafe for StringLit
impl Send for StringLit
impl Sync for StringLit
impl Unpin for StringLit
impl UnwindSafe for StringLit
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::StructDef
pub struct StructDef(pub Spanned<KeywordAndVisibility>, pub Spanned<Ident>, pub Block<Spanned<FieldDef>>);
Tuple Fields
0: Spanned<KeywordAndVisibility>``1: Spanned<Ident>``2: Block<Spanned<FieldDef>>
Trait Implementations
impl Clone for StructDef
fn clone(&self) -> StructDef
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for StructDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for StructDef
fn eq(&self, other: &StructDef) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for StructDef
Auto Trait Implementations
impl Freeze for StructDef
impl RefUnwindSafe for StructDef
impl Send for StructDef
impl Sync for StructDef
impl Unpin for StructDef
impl UnwindSafe for StructDef
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::Tuple
pub struct Tuple<T>(pub Vec<T>);
Tuple Fields
0: Vec<T>
Trait Implementations
impl<T: Clone> Clone for Tuple<T>
fn clone(&self) -> Tuple<T>
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl<T: Debug> Debug for Tuple<T>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl<T: PartialEq> PartialEq for Tuple<T>
fn eq(&self, other: &Tuple<T>) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl<T> StructuralPartialEq for Tuple<T>
Auto Trait Implementations
impl<T> Freeze for Tuple<T>
impl<T> RefUnwindSafe for Tuple<T>where T: RefUnwindSafe,
impl<T> Send for Tuple<T>where T: Send,
impl<T> Sync for Tuple<T>where T: Sync,
impl<T> Unpin for Tuple<T>where T: Unpin,
impl<T> UnwindSafe for Tuple<T>where T: UnwindSafe,
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::ast::UseDef
pub struct UseDef(pub Spanned<KeywordAndVisibility>, pub Vec<Spanned<Ident>>, pub Spanned<Ident>);
Tuple Fields
0: Spanned<KeywordAndVisibility>``1: Vec<Spanned<Ident>>``2: Spanned<Ident>
Trait Implementations
impl Clone for UseDef
fn clone(&self) -> UseDef
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for UseDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl Display for UseDef
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for UseDef
fn eq(&self, other: &UseDef) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for UseDef
Auto Trait Implementations
impl Freeze for UseDef
impl RefUnwindSafe for UseDef
impl Send for UseDef
impl Sync for UseDef
impl Unpin for UseDef
impl UnwindSafe for UseDef
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Module srclang::parser::span
Re-exports
pub use crate::lexer::Location;
:pub use crate::Db;
:
Structs
ByteOrLineColOrCoordInterned
:SourceMap
:Spanned
:__SourceMapConfig
:
Enums
ByteOrLineColOrCoord
:
Traits
HasChildSpans
:Spanning
:
Enum srclang::parser::span::ByteOrLineColOrCoord
pub enum ByteOrLineColOrCoord {
Byte(usize),
LineCol(usize, usize),
Coord(Location),
}
Variants
Byte(usize)
LineCol(usize, usize)
Coord(Location)
Trait Implementations
impl Clone for ByteOrLineColOrCoord
fn clone(&self) -> ByteOrLineColOrCoord
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for ByteOrLineColOrCoord
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl PartialEq for ByteOrLineColOrCoord
fn eq(&self, other: &ByteOrLineColOrCoord) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl StructuralPartialEq for ByteOrLineColOrCoord
Auto Trait Implementations
impl Freeze for ByteOrLineColOrCoord
impl RefUnwindSafe for ByteOrLineColOrCoord
impl Send for ByteOrLineColOrCoord
impl Sync for ByteOrLineColOrCoord
impl Unpin for ByteOrLineColOrCoord
impl UnwindSafe for ByteOrLineColOrCoord
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::span::ByteOrLineColOrCoordInterned
pub struct ByteOrLineColOrCoordInterned(/* private fields */);
Implementations
impl ByteOrLineColOrCoordInterned
pub fn new(__db: &<Jar as Jar<'_>>::DynDb, pos: ByteOrLineColOrCoord) -> Self
pub fn pos<'db>( self, __db: &'db <Jar as Jar<'_>>::DynDb) -> &'db ByteOrLineColOrCoord
pub fn set_pos<'db>( self, __db: &'db mut <Jar as Jar<'_>>::DynDb) -> Setter<'db, ByteOrLineColOrCoordInterned, ByteOrLineColOrCoord>
Trait Implementations
impl AsId for ByteOrLineColOrCoordInterned
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for ByteOrLineColOrCoordInterned
fn clone(&self) -> ByteOrLineColOrCoordInterned
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for ByteOrLineColOrCoordInterned
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for ByteOrLineColOrCoordInterned
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<ByteOrLineColOrCoordInterned> for Jar
fn ingredient( &self ) -> &<ByteOrLineColOrCoordInterned as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <ByteOrLineColOrCoordInterned as IngredientsFor>::Ingredients
impl Hash for ByteOrLineColOrCoordInterned
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for ByteOrLineColOrCoordInterned
type Jar = Jar
type Ingredients = (InputFieldIngredient<ByteOrLineColOrCoordInterned, ByteOrLineColOrCoord>, InputIngredient<ByteOrLineColOrCoordInterned>)
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for ByteOrLineColOrCoordInterned
fn cmp(&self, other: &ByteOrLineColOrCoordInterned) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for ByteOrLineColOrCoordInterned
fn eq(&self, other: &ByteOrLineColOrCoordInterned) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for ByteOrLineColOrCoordInterned
fn partial_cmp(&self, other: &ByteOrLineColOrCoordInterned) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for ByteOrLineColOrCoordInternedwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(_db: &DB, _index: IngredientIndex)
impl Copy for ByteOrLineColOrCoordInterned
impl Eq for ByteOrLineColOrCoordInterned
impl StructuralPartialEq for ByteOrLineColOrCoordInterned
Auto Trait Implementations
impl Freeze for ByteOrLineColOrCoordInterned
impl RefUnwindSafe for ByteOrLineColOrCoordInterned
impl Send for ByteOrLineColOrCoordInterned
impl Sync for ByteOrLineColOrCoordInterned
impl Unpin for ByteOrLineColOrCoordInterned
impl UnwindSafe for ByteOrLineColOrCoordInterned
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::parser::span::SourceMap
pub struct SourceMap(/* private fields */);
Implementations
impl SourceMap
pub fn new( __db: &<Jar as Jar<'_>>::DynDb, tokens: HashMap<Range<Location>, Node> ) -> Self
Trait Implementations
impl AsId for SourceMap
fn as_id(self) -> Id
fn from_id(id: Id) -> Self
impl Clone for SourceMap
fn clone(&self) -> SourceMap
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl Debug for SourceMap
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for SourceMap
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<SourceMap> for Jar
fn ingredient(&self) -> &<SourceMap as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <SourceMap as IngredientsFor>::Ingredients
impl Hash for SourceMap
fn hash<__H: Hasher>(&self, state: &mut __H)
Feeds this value into the given Hasher
. Read more1.3.0 ·
fn hash_slice<H>(data: &[Self], state: &mut H)where H: Hasher, Self: Sized,
Feeds a slice of this type into the given Hasher
. Read more
impl IngredientsFor for SourceMap
type Jar = Jar
type Ingredients = (TrackedStructIngredient<__SourceMapConfig>, [TrackedFieldIngredient<__SourceMapConfig>; 1])
fn create_ingredients<DB>(routes: &mut Routes<DB>) -> Self::Ingredientswhere DB: DbWithJar<Self::Jar> + JarFromJars<Self::Jar>,
impl Ord for SourceMap
fn cmp(&self, other: &SourceMap) -> Ordering
This method returns an Ordering
between self
and other
. Read more1.21.0 ·
fn max(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the maximum of two values. Read more1.21.0 ·
fn min(self, other: Self) -> Selfwhere Self: Sized,
Compares and returns the minimum of two values. Read more1.50.0 ·
fn clamp(self, min: Self, max: Self) -> Selfwhere Self: Sized + PartialOrd,
Restrict a value to a certain interval. Read more
impl PartialEq for SourceMap
fn eq(&self, other: &SourceMap) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl PartialOrd for SourceMap
fn partial_cmp(&self, other: &SourceMap) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<DB> SalsaStructInDb<DB> for SourceMapwhere DB: ?Sized + DbWithJar<Jar>,
fn register_dependent_fn(db: &DB, index: IngredientIndex)
impl<DB> TrackedStructInDb<DB> for SourceMapwhere DB: ?Sized + DbWithJar<Jar>,
fn database_key_index(self, db: &DB) -> DatabaseKeyIndex
Converts the identifier for this tracked struct into a DatabaseKeyIndex
.
impl Copy for SourceMap
impl Eq for SourceMap
impl StructuralPartialEq for SourceMap
Auto Trait Implementations
impl Freeze for SourceMap
impl RefUnwindSafe for SourceMap
impl Send for SourceMap
impl Sync for SourceMap
impl Unpin for SourceMap
impl UnwindSafe for SourceMap
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Comparable<K> for Qwhere Q: Ord + ?Sized, K: Borrow<Q> + ?Sized,
fn compare(&self, key: &K) -> Ordering
Compare self to key
and return their ordering.
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
impl<T> InputId for Twhere T: AsId,
impl<T> InternedData for Twhere T: Eq + Hash + Clone,
impl<T> InternedId for Twhere T: AsId,
Struct srclang::parser::span::Spanned
pub struct Spanned<T>(pub Location, pub T, pub Location);
Tuple Fields
0: Location``1: T``2: Location
Implementations
impl<T> Spanned<T>
pub fn span(&self) -> Range<Location>
pub fn span_size(&self) -> usize
impl<T> Spanned<T>
pub fn overlap(&self, other: &ByteOrLineColOrCoord) -> bool
Trait Implementations
impl<T: Clone> Clone for Spanned<T>
fn clone(&self) -> Spanned<T>
Returns a copy of the value. Read more1.0.0 ·
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from source
. Read more
impl<T: Debug> Debug for Spanned<T>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl<T: Display> Display for Spanned<T>
fn fmt(&self, f: &mut Formatter<'_>) -> Result
Formats the value using the given formatter. Read more
impl From<Spanned<Kw>> for Keyword
fn from(keyword: Spanned<Kw>) -> Self
Converts to this type from the input type.
impl<T: PartialEq> PartialEq for Spanned<T>
fn eq(&self, other: &Spanned<T>) -> bool
This method tests for self
and other
values to be equal, and is used by ==
.1.0.0 ·
fn ne(&self, other: &Rhs) -> bool
This method tests for !=
. The default implementation is almost always sufficient, and should not be overridden without very good reason.
impl<T: PartialOrd> PartialOrd for Spanned<T>
fn partial_cmp(&self, other: &Spanned<T>) -> Option<Ordering>
This method returns an ordering between self
and other
values if one exists. Read more1.0.0 ·
fn lt(&self, other: &Rhs) -> bool
This method tests less than (for self
and other
) and is used by the <
operator. Read more1.0.0 ·
fn le(&self, other: &Rhs) -> bool
This method tests less than or equal to (for self
and other
) and is used by the <=
operator. Read more1.0.0 ·
fn gt(&self, other: &Rhs) -> bool
This method tests greater than (for self
and other
) and is used by the >
operator. Read more1.0.0 ·
fn ge(&self, other: &Rhs) -> bool
This method tests greater than or equal to (for self
and other
) and is used by the >=
operator. Read more
impl<T: Sized + Send + Sync> Spanning for Spanned<T>
fn span(&self) -> Range<Location>
fn byte_range(&self) -> Range<usize>
fn line_col_range(&self) -> Range<(usize, usize)>
fn overlap(&self, other: &ByteOrLineColOrCoord) -> bool
impl<T: Eq> Eq for Spanned<T>
impl<T> StructuralPartialEq for Spanned<T>
Auto Trait Implementations
impl<T> Freeze for Spanned<T>where T: Freeze,
impl<T> RefUnwindSafe for Spanned<T>where T: RefUnwindSafe,
impl<T> Send for Spanned<T>where T: Send,
impl<T> Sync for Spanned<T>where T: Sync,
impl<T> Unpin for Spanned<T>where T: Unpin,
impl<T> UnwindSafe for Spanned<T>where T: UnwindSafe,
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Checks if this value is equivalent to the given key. Read more
impl<Q, K> Equivalent<K> for Qwhere Q: Eq + ?Sized, K: Borrow<Q> + ?Sized,
fn equivalent(&self, key: &K) -> bool
Compare self to key
and return true
if they are equal.
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T> ToOwned for Twhere T: Clone,
type Owned = T
The resulting type after obtaining ownership.
fn to_owned(&self) -> T
Creates owned data from borrowed data, usually by cloning. Read more
fn clone_into(&self, target: &mut T)
Uses borrowed data to replace owned data, usually by cloning. Read more
impl<T> ToString for Twhere T: Display + ?Sized,
default fn to_string(&self) -> String
Converts the given value to a String
. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Struct srclang::parser::span::__SourceMapConfig
pub struct __SourceMapConfig { /* private fields */ }
Trait Implementations
impl Configuration for __SourceMapConfig
type Id = SourceMap
The id type used to define instances of this struct. The TrackedStructIngredient
contains the interner that will create the id values.
type Fields = (HashMap<Range<Location>, Node>,)
A (possibly empty) tuple of the fields for this struct.
type Revisions = [Revision; 1]
A array of Revision
values, one per each of the value fields. When a struct is re-recreated in a new revision, the corresponding entries for each field are updated to the new revision if their values have changed (or if the field is marked as #[no_eq]
).
fn id_fields(fields: &Self::Fields) -> impl Hash
fn revision(revisions: &Self::Revisions, field_index: u32) -> Revision
Access the revision of a given value field.field_index
will be between 0 and the number of value fields.
fn new_revisions(current_revision: Revision) -> Self::Revisions
Create a new value revision array where each element is set to current_revision
.
fn update_revisions( current_revision_: Revision, old_value_: &Self::Fields, new_value_: &Self::Fields, revisions_: &mut Self::Revisions)
Update an existing value revision array revisions
, given the tuple of the old values (old_value
) and the tuple of the values (new_value
). If a value has changed, then its element is updated to current_revision
.
Auto Trait Implementations
impl Freeze for __SourceMapConfig
impl RefUnwindSafe for __SourceMapConfig
impl Send for __SourceMapConfig
impl Sync for __SourceMapConfig
impl Unpin for __SourceMapConfig
impl UnwindSafe for __SourceMapConfig
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Trait srclang::parser::span::HasChildSpans
pub trait HasChildSpanswhere
Self: Send + Sync + Sized + Spanning,{
// Required method
fn children(&self) -> impl IntoIterator<Item = Self>;
}
Required Methods
fn children(&self) -> impl IntoIterator<Item = Self>
Object Safety
This trait is not object safe.
Implementors
Trait srclang::parser::span::Spanning
pub trait Spanning: Send + Sync + Sized {
// Required method
fn span(&self) -> Range<Location>;
// Provided methods
fn byte_range(&self) -> Range<usize> { ... }
fn line_col_range(&self) -> Range<(usize, usize)> { ... }
fn overlap(&self, other: &ByteOrLineColOrCoord) -> bool { ... }
}
Required Methods
fn span(&self) -> Range<Location>
Provided Methods
fn byte_range(&self) -> Range<usize>
fn line_col_range(&self) -> Range<(usize, usize)>
fn overlap(&self, other: &ByteOrLineColOrCoord) -> bool
Object Safety
This trait is not object safe.
Implementations on Foreign Types
impl Spanning for &Range<Location>
fn span(&self) -> Range<Location>
Implementors
impl<T: Sized + Send + Sync> Spanning for Spanned<T>
Module srclang::parser::src
Structs
SourceParser
:
Traits
__ToTriple
:
Struct srclang::parser::src::SourceParser
pub struct SourceParser { /* private fields */ }
Implementations
impl SourceParser
pub fn new() -> SourceParser
pub fn parse<'input, 'err, __TOKEN: __ToTriple<'input, 'err>, __TOKENS: IntoIterator<Item = __TOKEN>>( &self, errors: &'err mut Vec<ErrorRecovery<Location, Token<'input>, &'static str>>, db: &dyn Db, __tokens0: __TOKENS ) -> Result<Module, ParseError<Location, Token<'input>, &'static str>>
Trait Implementations
impl Default for SourceParser
fn default() -> Self
Returns the “default value” for a type. Read more
Auto Trait Implementations
impl Freeze for SourceParser
impl RefUnwindSafe for SourceParser
impl Send for SourceParser
impl Sync for SourceParser
impl Unpin for SourceParser
impl UnwindSafe for SourceParser
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Trait srclang::parser::src::__ToTriple
pub trait __ToTriple<'input, 'err> {
// Required method
fn to_triple(
value: Self
) -> Result<(Location, Token<'input>, Location), ParseError<Location, Token<'input>, &'static str>>;
}
Required Methods
fn to_triple( value: Self ) -> Result<(Location, Token<'input>, Location), ParseError<Location, Token<'input>, &'static str>>
Object Safety
This trait is not object safe.
Implementations on Foreign Types
impl<'input, 'err> __ToTriple<'input, 'err> for (Location, Token<'input>, Location)
fn to_triple( value: Self ) -> Result<(Location, Token<'input>, Location), ParseError<Location, Token<'input>, &'static str>>
impl<'input, 'err> __ToTriple<'input, 'err> for Result<(Location, Token<'input>, Location), &'static str>
fn to_triple( value: Self ) -> Result<(Location, Token<'input>, Location), ParseError<Location, Token<'input>, &'static str>>
Implementors
Struct srclang::Jar
pub struct Jar(/* private fields */);
The salsa database for the compiler. The default convention is to implement this at the crate level.
Trait Implementations
impl DbWithJar<Jar> for Database
fn as_jar_db<'db>(&'db self) -> &'db <Jar as Jar<'db>>::DynDbwhere 'db: 'db,
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for ByteOrLineColOrCoordInterned
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Document
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for EffectDef
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Function
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Import
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for InternedEffect
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Mangled
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Position
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Program
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for SourceMap
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for SourceMap
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for SourceProgram
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Span
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Spanned
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Symbol
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for SyntaxTree
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl DebugWithDb<<Jar as Jar<'_>>::DynDb> for Url
fn fmt(&self, f: &mut Formatter<'_>, _db: &<Jar as Jar<'_>>::DynDb) -> Result
Format self
given the database db
. Read more
fn debug<'me, 'db>(&'me self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
fn into_debug<'me, 'db>(self, db: &'me Db) -> DebugWith<'me, Db>where Self: Sized + 'me,
Creates a wrapper type that implements Debug
but which uses the DebugWithDb::fmt
. Read more
impl HasIngredientsFor<ByteOrLineColOrCoordInterned> for Jar
fn ingredient( &self ) -> &<ByteOrLineColOrCoordInterned as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <ByteOrLineColOrCoordInterned as IngredientsFor>::Ingredients
impl HasIngredientsFor<Document> for Jar
fn ingredient(&self) -> &<Document as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Document as IngredientsFor>::Ingredients
impl HasIngredientsFor<EffectDef> for Jar
fn ingredient(&self) -> &<EffectDef as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <EffectDef as IngredientsFor>::Ingredients
impl HasIngredientsFor<Function> for Jar
fn ingredient(&self) -> &<Function as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Function as IngredientsFor>::Ingredients
impl HasIngredientsFor<Import> for Jar
fn ingredient(&self) -> &<Import as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Import as IngredientsFor>::Ingredients
impl HasIngredientsFor<InternedEffect> for Jar
fn ingredient(&self) -> &<InternedEffect as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <InternedEffect as IngredientsFor>::Ingredients
impl HasIngredientsFor<Mangled> for Jar
fn ingredient(&self) -> &<Mangled as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Mangled as IngredientsFor>::Ingredients
impl HasIngredientsFor<Position> for Jar
fn ingredient(&self) -> &<Position as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Position as IngredientsFor>::Ingredients
impl HasIngredientsFor<Program> for Jar
fn ingredient(&self) -> &<Program as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Program as IngredientsFor>::Ingredients
impl HasIngredientsFor<SourceMap> for Jar
fn ingredient(&self) -> &<SourceMap as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <SourceMap as IngredientsFor>::Ingredients
impl HasIngredientsFor<SourceMap> for Jar
fn ingredient(&self) -> &<SourceMap as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <SourceMap as IngredientsFor>::Ingredients
impl HasIngredientsFor<SourceProgram> for Jar
fn ingredient(&self) -> &<SourceProgram as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <SourceProgram as IngredientsFor>::Ingredients
impl HasIngredientsFor<Span> for Jar
fn ingredient(&self) -> &<Span as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Span as IngredientsFor>::Ingredients
impl HasIngredientsFor<Spanned> for Jar
fn ingredient(&self) -> &<Spanned as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Spanned as IngredientsFor>::Ingredients
impl HasIngredientsFor<Symbol> for Jar
fn ingredient(&self) -> &<Symbol as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Symbol as IngredientsFor>::Ingredients
impl HasIngredientsFor<SyntaxTree> for Jar
fn ingredient(&self) -> &<SyntaxTree as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <SyntaxTree as IngredientsFor>::Ingredients
impl HasIngredientsFor<Url> for Jar
fn ingredient(&self) -> &<Url as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <Url as IngredientsFor>::Ingredients
impl HasIngredientsFor<add_file> for Jar
fn ingredient(&self) -> &<add_file as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <add_file as IngredientsFor>::Ingredients
impl HasIngredientsFor<add_imports> for Jar
fn ingredient(&self) -> &<add_imports as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <add_imports as IngredientsFor>::Ingredients
impl HasIngredientsFor<calculate_line_lengths> for Jar
fn ingredient(&self) -> &<calculate_line_lengths as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <calculate_line_lengths as IngredientsFor>::Ingredients
impl HasIngredientsFor<compile> for Jar
fn ingredient(&self) -> &<compile as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <compile as IngredientsFor>::Ingredients
impl HasIngredientsFor<compile_effect> for Jar
fn ingredient(&self) -> &<compile_effect as IngredientsFor>::Ingredients
fn ingredient_mut( &mut self ) -> &mut <compile_effect as IngredientsFor>::Ingredients
impl HasIngredientsFor<get_symbol> for Jar
fn ingredient(&self) -> &<get_symbol as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <get_symbol as IngredientsFor>::Ingredients
impl HasIngredientsFor<span_text> for Jar
fn ingredient(&self) -> &<span_text as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <span_text as IngredientsFor>::Ingredients
impl HasIngredientsFor<to_spans> for Jar
fn ingredient(&self) -> &<to_spans as IngredientsFor>::Ingredients
fn ingredient_mut(&mut self) -> &mut <to_spans as IngredientsFor>::Ingredients
impl HasJar<Jar> for Database
fn jar(&self) -> (&Jar, &Runtime)
fn jar_mut(&mut self) -> (&mut Jar, &mut Runtime)
impl<'salsa_db> Jar<'salsa_db> for Jar
type DynDb = dyn Db + 'salsa_db
unsafe fn init_jar<DB>(place: *mut Self, routes: &mut Routes<DB>)where DB: JarFromJars<Self> + DbWithJar<Self>,
Initializes the jar at place
Read more
impl JarFromJars<Jar> for Database
fn jar_from_jars<'db>(jars: &Self::Jars) -> &Jar
fn jar_from_jars_mut<'db>(jars: &mut Self::Jars) -> &mut Jar
Auto Trait Implementations
impl !Freeze for Jar
impl !RefUnwindSafe for Jar
impl Send for Jar
impl Sync for Jar
impl Unpin for Jar
impl !UnwindSafe for Jar
Blanket Implementations
impl<T> Any for Twhere T: 'static + ?Sized,
fn type_id(&self) -> TypeId
Gets the TypeId
of self
. Read more
impl<T> Borrow<T> for Twhere T: ?Sized,
fn borrow(&self) -> &T
Immutably borrows from an owned value. Read more
impl<T> BorrowMut<T> for Twhere T: ?Sized,
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
impl<T> From<T> for T
fn from(t: T) -> T
Returns the argument unchanged.
impl<T, U> Into<U> for Twhere U: From<T>,
fn into(self) -> U
Calls U::from(self)
.
That is, this conversion is whatever the implementation of From<T> for U
chooses to do.
impl<T> Pointable for T
const ALIGN: usize = _
The alignment of pointer.
type Init = T
The type for initializers.
unsafe fn init(init: <T as Pointable>::Init) -> usize
Initializes a with the given initializer. Read more
unsafe fn deref<'a>(ptr: usize) -> &'a T
Dereferences the given pointer. Read more
unsafe fn deref_mut<'a>(ptr: usize) -> &'a mut T
Mutably dereferences the given pointer. Read more
unsafe fn drop(ptr: usize)
Drops the object pointed to by the given pointer. Read more
impl<T, U> TryFrom<U> for Twhere U: Into<T>,
type Error = Infallible
The type returned in the event of a conversion error.
fn try_from(value: U) -> Result<T, <T as TryFrom<U>>::Error>
Performs the conversion.
impl<T, U> TryInto<U> for Twhere U: TryFrom<T>,
type Error = <U as TryFrom<T>>::Error
The type returned in the event of a conversion error.
fn try_into(self) -> Result<U, <U as TryFrom<T>>::Error>
Performs the conversion.
Trait srclang::Db
pub trait Db: DbWithJar<Jar> { }
The Db trait is a marker trait that is used to ensure that the Jar struct is a valid salsa database. The default convention is to implement this at the crate level.
Implementors
impl<DB> Db for DBwhere DB: ?Sized + DbWithJar<Jar>,
Implement the Db trait for the Jar struct. The default convention is to implement this at the crate level.
Crate srclang_derive
Attribute Macros
node
: Defines a node.
Attribute Macro srclang_derive::node
#[node]
Defines a node.ⓘ
#[node]
struct MyNode {
field: Type,
}
which will expand to:ⓘ
struct MyNode {
field: Spanned<Type>,
}